Using the Naive Bayes Classifier (also supervised)¶
(This notebook was created for a workshop at RV College of Engineering on 6th Sep 2021)
Consider an example :
X is a 30 year old customer who wants to buy a computer.
His salary is Rs. 80000
Here age and salary are 2 attributes - x1
and x2
.
Let’s say H
is the hypothesis that a customer will buy a computer. And C
is the class of Computer buyers.
We want to find the probability of X belonging to C
i.e P(H|X)
- termed A Posteriori Probability
Here, the probability that any customer regardless of age or salary purchasing a computer is P(H)
- the A Priori Probability of a computer being purchased
Similarly,
P(X|H)
is the A posteriori probability of X conditioned on H. That is, it is the probability of a customer X being 30 years old and earning Rs.80000, given that we know X will buy a computer.
P(X) is the A priori probability of X being 30 years old and earning Rs.80000.
According to Bayes’ theorem, the probability that we want to compute P(H|X)
can be expressed in terms of probabilities P(H), P(X|H), and P(X) as
Terms learnt :
A Priori Probability
Hypothesis
A Posteriori Probability
Likelihood
The data samples are described by attributes age, income and student. The BuyClass label attribute, buy, tells whether the person buys a computer, has two distinct values, 1 (class C1) and 0 (class C2). The sample we want to classify i.e. test is X = (age = A, income = H, student = 0) We need to maximize P(X|Ci)P(Ci), for i = 1, 2.
P(Ci), the A Priori probability of each class, can be estimated based on the training samples:
P(buy = 1) = 9/14
P(buy = 0) = 5/14
To compute P(X|Ci), for i = 1, 2, we compute the following conditional probabilities:
P(age = A|buy = yes)
\(= 6/9\)P(age = A|buy = no)
\(= 1/5\)P(income = H|buy = yes)
\(= 4/9\)P(income = H|buy = no)
\(= 0/5\)P(student = 0|buy = yes)
\(= 6/9\)P(student = 0|buy = no)
\(=2/5\)
Using the above probabilities, we obtain
P(X|buy = yes) =
\(P(age = A|buy = yes) * P(income = H|buy = yes) * P(student = 0|buy = yes)\) = \((6/9) * (4/9) * (6/9)\) = 324/729 = 0.44
P(X|buy = no) = 0
To find the class that Maximizes P(X|Ci)P(Ci), we compute
\(P(X|buy = yes) * P(buy = yes) = 0.44 * (9/14) = 0.28\)
\(P(X|buy = no) * P(buy = no) = 0 * (5/14) = 0\)
Therefore, the Naive Bayes Classifier predicts that the Man who is an Adult, with a high income and is not a student will buy a Computer.
Install libraries¶
[ ]:
# install nltk
!pip install nltk
!pip install pandas
# install gensim
!pip install gensim
!pip install seaborn
!pip install scikit-learn
# install wordcloud
!pip install wordcloud
Requirement already satisfied: nltk in /usr/local/lib/python3.8/dist-packages (3.6.2)
Requirement already satisfied: joblib in /usr/local/lib/python3.8/dist-packages (from nltk) (1.0.1)
Requirement already satisfied: click in /usr/local/lib/python3.8/dist-packages (from nltk) (8.0.1)
Requirement already satisfied: regex in /usr/local/lib/python3.8/dist-packages (from nltk) (2021.8.28)
Requirement already satisfied: tqdm in /usr/local/lib/python3.8/dist-packages (from nltk) (4.62.2)
WARNING: You are using pip version 20.3.3; however, version 21.2.4 is available.
You should consider upgrading via the '/usr/bin/python -m pip install --upgrade pip' command.
Requirement already satisfied: pandas in /usr/local/lib/python3.8/dist-packages (1.3.3)
Requirement already satisfied: python-dateutil>=2.7.3 in /usr/local/lib/python3.8/dist-packages (from pandas) (2.8.1)
Requirement already satisfied: numpy>=1.17.3 in /usr/local/lib/python3.8/dist-packages (from pandas) (1.21.2)
Requirement already satisfied: pytz>=2017.3 in /usr/local/lib/python3.8/dist-packages (from pandas) (2021.1)
Requirement already satisfied: six>=1.5 in /usr/lib/python3/dist-packages (from python-dateutil>=2.7.3->pandas) (1.14.0)
WARNING: You are using pip version 20.3.3; however, version 21.2.4 is available.
You should consider upgrading via the '/usr/bin/python -m pip install --upgrade pip' command.
Requirement already satisfied: gensim in /usr/local/lib/python3.8/dist-packages (4.1.0)
Requirement already satisfied: numpy>=1.17.0 in /usr/local/lib/python3.8/dist-packages (from gensim) (1.21.2)
Requirement already satisfied: scipy>=0.18.1 in /usr/local/lib/python3.8/dist-packages (from gensim) (1.7.1)
Requirement already satisfied: smart-open>=1.8.1 in /usr/local/lib/python3.8/dist-packages (from gensim) (5.2.1)
WARNING: You are using pip version 20.3.3; however, version 21.2.4 is available.
You should consider upgrading via the '/usr/bin/python -m pip install --upgrade pip' command.
Requirement already satisfied: seaborn in /usr/local/lib/python3.8/dist-packages (0.11.2)
Requirement already satisfied: matplotlib>=2.2 in /usr/local/lib/python3.8/dist-packages (from seaborn) (3.4.3)
Requirement already satisfied: numpy>=1.15 in /usr/local/lib/python3.8/dist-packages (from seaborn) (1.21.2)
Requirement already satisfied: pandas>=0.23 in /usr/local/lib/python3.8/dist-packages (from seaborn) (1.3.3)
Requirement already satisfied: scipy>=1.0 in /usr/local/lib/python3.8/dist-packages (from seaborn) (1.7.1)
Requirement already satisfied: python-dateutil>=2.7 in /usr/local/lib/python3.8/dist-packages (from matplotlib>=2.2->seaborn) (2.8.1)
Requirement already satisfied: cycler>=0.10 in /usr/local/lib/python3.8/dist-packages (from matplotlib>=2.2->seaborn) (0.10.0)
Requirement already satisfied: pyparsing>=2.2.1 in /usr/local/lib/python3.8/dist-packages (from matplotlib>=2.2->seaborn) (2.4.7)
Requirement already satisfied: kiwisolver>=1.0.1 in /usr/local/lib/python3.8/dist-packages (from matplotlib>=2.2->seaborn) (1.3.2)
Requirement already satisfied: pillow>=6.2.0 in /usr/local/lib/python3.8/dist-packages (from matplotlib>=2.2->seaborn) (8.3.2)
Requirement already satisfied: six in /usr/lib/python3/dist-packages (from cycler>=0.10->matplotlib>=2.2->seaborn) (1.14.0)
Requirement already satisfied: pytz>=2017.3 in /usr/local/lib/python3.8/dist-packages (from pandas>=0.23->seaborn) (2021.1)
WARNING: You are using pip version 20.3.3; however, version 21.2.4 is available.
You should consider upgrading via the '/usr/bin/python -m pip install --upgrade pip' command.
Requirement already satisfied: scikit-learn in /usr/local/lib/python3.8/dist-packages (0.24.2)
Requirement already satisfied: threadpoolctl>=2.0.0 in /usr/local/lib/python3.8/dist-packages (from scikit-learn) (2.2.0)
Requirement already satisfied: scipy>=0.19.1 in /usr/local/lib/python3.8/dist-packages (from scikit-learn) (1.7.1)
Requirement already satisfied: joblib>=0.11 in /usr/local/lib/python3.8/dist-packages (from scikit-learn) (1.0.1)
Requirement already satisfied: numpy>=1.13.3 in /usr/local/lib/python3.8/dist-packages (from scikit-learn) (1.21.2)
WARNING: You are using pip version 20.3.3; however, version 21.2.4 is available.
You should consider upgrading via the '/usr/bin/python -m pip install --upgrade pip' command.
Requirement already satisfied: wordcloud in /usr/local/lib/python3.8/dist-packages (1.8.1)
Requirement already satisfied: numpy>=1.6.1 in /usr/local/lib/python3.8/dist-packages (from wordcloud) (1.21.2)
Requirement already satisfied: matplotlib in /usr/local/lib/python3.8/dist-packages (from wordcloud) (3.4.3)
Requirement already satisfied: pillow in /usr/local/lib/python3.8/dist-packages (from wordcloud) (8.3.2)
Requirement already satisfied: cycler>=0.10 in /usr/local/lib/python3.8/dist-packages (from matplotlib->wordcloud) (0.10.0)
Requirement already satisfied: pyparsing>=2.2.1 in /usr/local/lib/python3.8/dist-packages (from matplotlib->wordcloud) (2.4.7)
Requirement already satisfied: python-dateutil>=2.7 in /usr/local/lib/python3.8/dist-packages (from matplotlib->wordcloud) (2.8.1)
Requirement already satisfied: kiwisolver>=1.0.1 in /usr/local/lib/python3.8/dist-packages (from matplotlib->wordcloud) (1.3.2)
Requirement already satisfied: six in /usr/lib/python3/dist-packages (from cycler>=0.10->matplotlib->wordcloud) (1.14.0)
WARNING: You are using pip version 20.3.3; however, version 21.2.4 is available.
You should consider upgrading via the '/usr/bin/python -m pip install --upgrade pip' command.
[ ]:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from wordcloud import WordCloud, STOPWORDS
import nltk
from nltk.stem import PorterStemmer, WordNetLemmatizer
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize, sent_tokenize
import gensim
from gensim.utils import simple_preprocess
from gensim.parsing.preprocessing import STOPWORDS
from sklearn.metrics import classification_report, confusion_matrix
[ ]:
resume_df = pd.read_csv("https://raw.githubusercontent.com/abhiramr/ML_Workshop_RVCE/main/naive_bayes/resume.csv", encoding='latin-1')
[ ]:
resume_df.shape
(125, 3)
[ ]:
# data containing resume
resume_df[["resume_text","class"]]
resume_df = resume_df[["class","resume_text"]]
[ ]:
# Question - Print the top 5 and last 5 rows of the dataframe
resume_df.iloc[0:5,-5:-1]
class | |
---|---|
0 | not_flagged |
1 | not_flagged |
2 | not_flagged |
3 | not_flagged |
4 | flagged |
[ ]:
resume_df.iloc[[0,]] #append
class | resume_text | |
---|---|---|
0 | not_flagged | \rCustomer Service Supervisor/Tier - Isabella ... |
Perform EDA¶
[ ]:
# obtain dataframe information
resume_df.info()
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 125 entries, 0 to 124
Data columns (total 2 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 class 125 non-null object
1 resume_text 125 non-null object
dtypes: object(2)
memory usage: 2.1+ KB
[ ]:
# check for null values
resume_df.isnull().sum()
class 0
resume_text 0
dtype: int64
[ ]:
resume_df['class'].value_counts()
not_flagged 92
flagged 33
Name: class, dtype: int64
[ ]:
resume_df["class"] = resume_df["class"].apply(lambda i:1 if i=="flagged" else 0)
[ ]:
# Question! - Can you divide the DataFrame into two smaller DFs? - One that belongs to class 0 and one that belongs to class 1.
resume_df1 = resume_df[resume_df['class']==1]
resume_df1.value_counts()
Perform Data Cleaning¶
resume_df['resume_text'].head()
[ ]:
resume_df['resume_text'] = resume_df['resume_text'].apply(lambda x: x.replace('\r',''))
[ ]:
resume_df['resume_text'].head()
0 Customer Service Supervisor/Tier - Isabella Ca...
1 Engineer / Scientist - IBM Microelectronics Di...
2 LTS Software Engineer Computational Lithograph...
3 TUTORWilliston VT - Email me on Indeed: indee...
4 Independent Consultant - Self-employedBurlingt...
Name: resume_text, dtype: object
[ ]:
# download nltk packages
nltk.download('punkt')
nltk.download("stopwords")
[nltk_data] Downloading package punkt to /root/nltk_data...
[nltk_data] Package punkt is already up-to-date!
[nltk_data] Downloading package stopwords to /root/nltk_data...
[nltk_data] Package stopwords is already up-to-date!
True
[ ]:
# Get additional stopwords from nltk
from nltk.corpus import stopwords
stop_words = stopwords.words('english')
[ ]:
# Remove stop words and remove words with 2 or less characters
def preprocess(text):
result = []
for token in gensim.utils.simple_preprocess(text) :
if token not in gensim.parsing.preprocessing.STOPWORDS and len(token) > 2 and token not in stop_words:
result.append(token)
return ' '.join(result)
[ ]:
# Cleaned text into a new column
resume_df["cleaned"] = resume_df["resume_text"].apply(preprocess)
Visualize Cleaned Dataset¶
[ ]:
# Plot the counts of flagged vs not flagged
sns.countplot(resume_df["class"], label = "Count Plot")
/usr/local/lib/python3.8/dist-packages/seaborn/_decorators.py:36: FutureWarning: Pass the following variable as a keyword arg: x. From version 0.12, the only valid positional argument will be `data`, and passing other arguments without an explicit keyword will result in an error or misinterpretation.
warnings.warn(
<AxesSubplot:xlabel='class', ylabel='count'>
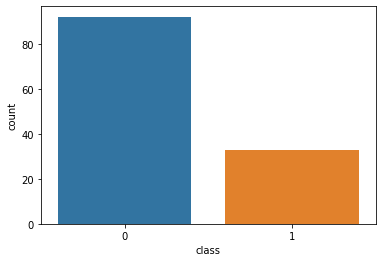
[ ]:
# plot the word cloud for text that is flagged
plt.figure(figsize = (20,20))
wc = WordCloud(max_words=2000, width=1600, height=800, stopwords=stop_words).generate(str(resume_df[resume_df['class']==1].cleaned))
plt.imshow(wc)
<matplotlib.image.AxesImage at 0x7f199232cd30>
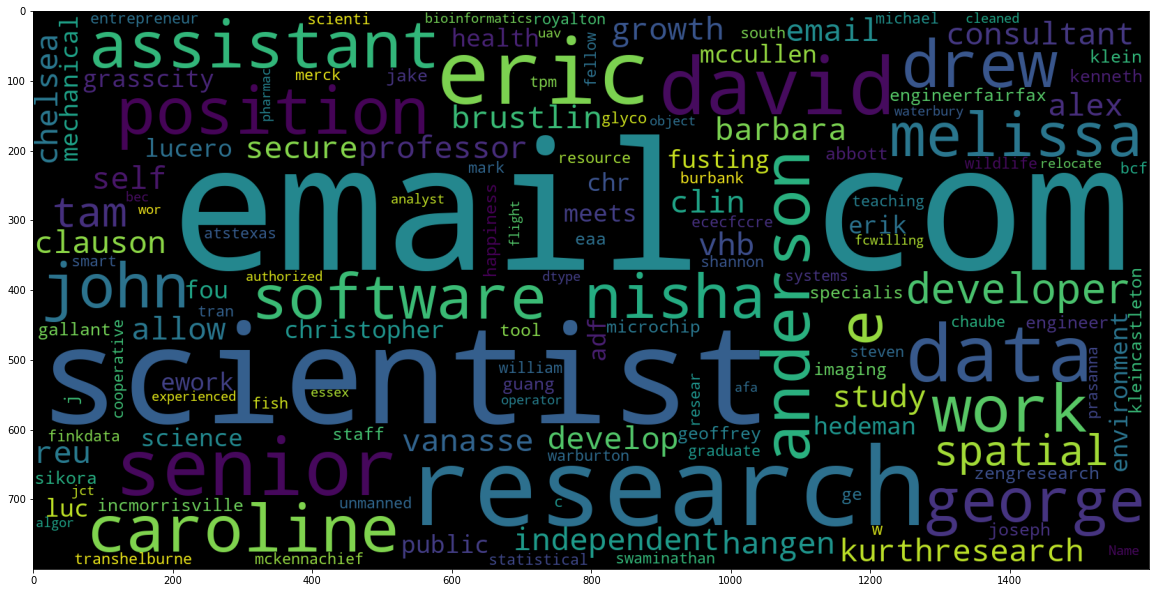
[ ]:
# Question - Plot the wordcloud for class 0
Prepare the Data by Applying Count Tokenizer¶
[ ]:
# CountVectorizer example
from sklearn.feature_extraction.text import CountVectorizer
sample_data = ['This is the first document.','This document is the second document.','And this is the third one.','Is this the first document?']
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(sample_data)
[ ]:
print(vectorizer.get_feature_names())
print(X.toarray())
['and', 'document', 'first', 'is', 'one', 'second', 'the', 'third', 'this']
[[0 1 1 1 0 0 1 0 1]
[0 2 0 1 0 1 1 0 1]
[1 0 0 1 1 0 1 1 1]
[0 1 1 1 0 0 1 0 1]]
[ ]:
# Applying CountVectorier to the cleaned text
vectorizer = CountVectorizer()
c = vectorizer.fit_transform(resume_df['cleaned'])
print(vectorizer.get_feature_names())
print(c.toarray())
#pd.set_option('display.max_cols', 50000)
['aaalac', 'aabb', 'aac', 'aacn', 'aacr', 'aacrjournals', 'aakeroõ_y', 'aanpcp', 'aaron', 'abbott', 'abdomen', 'abdominal', 'abdul', 'aberdeen', 'abi', 'abilities', 'ability', 'abiotic', 'able', 'abnormal', 'aboard', 'abosalem', 'abraham', 'abreast', 'abs', 'absence', 'absorbance', 'abstract', 'abstracta', 'abstractdisease', 'abstracted', 'abstractin', 'abstracts', 'abualrub', 'abundance', 'abureehan', 'abuse', 'abusiness', 'academia', 'academic', 'academics', 'academy', 'acaeefbc', 'accelerated', 'accept', 'acceptability', 'acceptable', 'acceptance', 'accepted', 'accepting', 'access', 'accessibility', 'accessible', 'accession', 'accident', 'accidents', 'accolateî', 'accommodations', 'accomplished', 'accomplishment', 'accomplishments', 'accord', 'accordance', 'according', 'accordingly', 'accords', 'account', 'accountability', 'accountable', 'accounted', 'accounting', 'accounts', 'accreditation', 'accredited', 'accrual', 'accumulation', 'accuracy', 'accurate', 'accurately', 'accustomed', 'acetylsalicylic', 'acf', 'acg', 'acheived', 'achieve', 'achieved', 'achievement', 'achievements', 'achieving', 'acid', 'acknowledgment', 'acknowledgments', 'acls', 'aco', 'aconsistent', 'acoustic', 'acoustics', 'acousticschief', 'acousticscto', 'acquire', 'acquired', 'acquiring', 'acquisition', 'acquisitions', 'acr', 'acre', 'acrylamide', 'acs', 'act', 'acted', 'acting', 'action', 'actions', 'activate', 'activation', 'active', 'actively', 'activities', 'activitieso', 'activity', 'acton', 'actor', 'actors', 'acts', 'actuated', 'actuators', 'acumen', 'acustomer', 'acute', 'acwestford', 'acwork', 'ada', 'adaboost', 'adapt', 'adaptability', 'adaptable', 'adaptation', 'adaptations', 'adapted', 'adapting', 'adaption', 'adaptive', 'adb', 'adcc', 'add', 'added', 'adding', 'addison', 'addition', 'additional', 'additionally', 'additions', 'additives', 'address', 'addressed', 'addresses', 'addressing', 'adenovirus', 'adept', 'adequate', 'adfe', 'adhere', 'adherence', 'adhering', 'adifferent', 'adjunct', 'adjust', 'adjusted', 'adjuster', 'adjustereberl', 'adjusters', 'adjusting', 'adjustments', 'adjuvanted', 'adjuvants', 'admin', 'administer', 'administered', 'administering', 'administration', 'administratione', 'administrative', 'administrator', 'administrators', 'admission', 'admissions', 'adn', 'adobe', 'adobeîâ', 'adolescent', 'adolescents', 'adopt', 'adopted', 'adopters', 'adoption', 'adoptions', 'adp', 'adt', 'adult', 'adults', 'advance', 'advanced', 'advancement', 'advancements', 'advancing', 'advantage', 'advantages', 'advantest', 'adventist', 'adventures', 'adverse', 'advertised', 'advertising', 'advia', 'advice', 'advised', 'advisement', 'adviser', 'adviserag', 'adviseressex', 'advisermcginn', 'adviserstifel', 'advising', 'advisor', 'advisorbp', 'advisors', 'advisory', 'advocate', 'advocating', 'aecf', 'aed', 'aeration', 'aerial', 'aero', 'aerobic', 'aerodynamic', 'aerodynamics', 'aerojet', 'aeromechanics', 'aeropropulsion', 'aerospace', 'aeroxchange', 'afa', 'affairs', 'affairswhite', 'affarsamerican', 'affect', 'affecting', 'affiliation', 'affiliations', 'affinity', 'affirm', 'affymetrix', 'afm', 'africa', 'african', 'afs', 'aftermath', 'afto', 'agar', 'agarose', 'agc', 'agchem', 'age', 'aged', 'agencies', 'agency', 'agent', 'agentmarketing', 'agents', 'agentsecond', 'agentvermander', 'ages', 'agewise', 'agg', 'aggregate', 'aggregated', 'aggregates', 'aggregatibacter', 'aggregation', 'aggressive', 'agile', 'agilent', 'agilents', 'agility', 'aging', 'agitated', 'agnostics', 'agonist', 'agonists', 'agreement', 'agreements', 'agricultural', 'agriculture', 'agroecology', 'agroforestry', 'agron', 'aha', 'ahead', 'ahima', 'aid', 'aidadditional', 'aide', 'aided', 'aiding', 'aids', 'aim', 'aimed', 'aimeerblair', 'aims', 'aimsweb', 'air', 'airborne', 'aircom', 'aircraft', 'airfoil', 'airpollution', 'airport', 'aix', 'ajax', 'ajplung', 'ajtmh', 'akron', 'alabama', 'alajuela', 'alamos', 'alarm', 'alaska', 'albans', 'albany', 'albedo', 'albert', 'alberta', 'albuquerque', 'alcohol', 'alec', 'alert', 'alerting', 'alex', 'alexander', 'alford', 'algae', 'algebra', 'algorithm', 'algorithmic', 'algorithms', 'algorithmsvideo', 'alice', 'align', 'aligned', 'alignment', 'alignments', 'alimentaria', 'aliquot', 'aliquoted', 'alison', 'alkalinity', 'allarm', 'alleged', 'allen', 'allergic', 'allergy', 'alleviate', 'alliance', 'allianceumass', 'allington', 'allocate', 'allocation', 'allotments', 'allow', 'allowed', 'allowing', 'allows', 'alloys', 'allscripts', 'allthings', 'almy', 'alongside', 'alpha', 'alphabetized', 'alteration', 'altered', 'alternative', 'alternatives', 'alto', 'alum', 'aluminum', 'alumni', 'alvin', 'alvinwhite', 'amateur', 'amazon', 'ambient', 'ambition', 'ambitious', 'ambulating', 'ambulation', 'america', 'american', 'americana', 'americas', 'amerivan', 'amherst', 'ammonia', 'ammunition', 'amoco', 'amounts', 'amphetamines', 'amphibian', 'amphibians', 'amplicon', 'amplicons', 'amplification', 'amplified', 'amplifiers', 'amt', 'amtrak', 'anabat', 'anadromous', 'anaerobic', 'analog', 'analyses', 'analysis', 'analysisallen', 'analysiscontrol', 'analysiscurrent', 'analysiso', 'analysisskills', 'analysisslide', 'analysissoil', 'analyst', 'analystkeurig', 'analystnasa', 'analysto', 'analystolympus', 'analystpbm', 'analystresource', 'analystrocket', 'analysts', 'analystshort', 'analystwil', 'analytes', 'analytic', 'analytical', 'analytics', 'analyze', 'analyzed', 'analyzer', 'analyzers', 'analyzing', 'anational', 'anatomy', 'anaylsis', 'anchorage', 'ancient', 'anco', 'andanalysis', 'andatmospheric', 'andcanada', 'andcritical', 'andcustom', 'andean', 'anderson', 'andgeneral', 'andhabitats', 'andnormal', 'andpneumatics', 'andprovided', 'android', 'androidmanaging', 'androscoggin', 'andwork', 'andwriting', 'anearly', 'anechoic', 'anesthesia', 'anesthetic', 'anesthetized', 'aneurysm', 'anever', 'anew', 'angel', 'angeles', 'angiography', 'angstrom', 'angular', 'angularjs', 'anhydrous', 'animal', 'animals', 'animated', 'animation', 'anionic', 'anjou', 'ankle', 'ann', 'anneal', 'anneals', 'annexin', 'anniversary', 'annotations', 'annual', 'annually', 'anomaly', 'anorad', 'anova', 'answer', 'answered', 'answering', 'ant', 'antand', 'antarctic', 'antarctica', 'antenna', 'anti', 'antibiotic', 'antibiotics', 'antibodies', 'antibody', 'anticancer', 'anticipate', 'anticipated', 'antigen', 'antigens', 'antimalarials', 'antimicrobial', 'antinuclear', 'antonio', 'anxiety', 'anywherework', 'aortic', 'aox', 'apa', 'apache', 'apath', 'aphaenogaster', 'api', 'apical', 'apis', 'apoptosis', 'apothecary', 'app', 'appaloosa', 'appeal', 'appearing', 'appendices', 'appl', 'apple', 'apples', 'appletalk', 'applicability', 'applicable', 'applicants', 'application', 'applicationo', 'applications', 'applicationso', 'applied', 'apply', 'applying', 'appointed', 'appointments', 'appraisal', 'appraising', 'appreciation', 'apprentice', 'apprenticeship', 'apprenticevt', 'approach', 'approaches', 'approaching', 'appropriate', 'appropriately', 'approval', 'approvals', 'approvalthese', 'approve', 'approved', 'approves', 'approximately', 'apps', 'apr', 'april', 'aprn', 'aproblem', 'aptitude', 'aqlm', 'aquaculture', 'aquarist', 'aquarium', 'aquariums', 'aquatic', 'aquifer', 'arab', 'arabesque', 'arabian', 'aramco', 'arametric', 'arbotics', 'arc', 'arcedit', 'arcgis', 'archaeological', 'architect', 'architected', 'architecture', 'archival', 'archive', 'arcing', 'arcpad', 'arctic', 'ard', 'arduino', 'area', 'areas', 'arena', 'arenas', 'arerugged', 'ares', 'argue', 'argument', 'argus', 'aricent', 'arise', 'arizona', 'arlg', 'arlington', 'arm', 'armonk', 'armory', 'armstrong', 'army', 'armypropulsion', 'arnoletti', 'aromatic', 'aromatics', 'arose', 'arra', 'arrange', 'arranged', 'arrangements', 'arranging', 'array', 'arrays', 'arresting', 'arrival', 'arrive', 'arrived', 'arroyo', 'ars', 'arsenal', 'arsenic', 'art', 'artappreciation', 'arteries', 'artery', 'arthur', 'artic', 'article', 'articles', 'articulate', 'articulating', 'artifacts', 'artificial', 'artist', 'artistcharles', 'artistic', 'artistjager', 'artists', 'arts', 'artscompeted', 'artssaint', 'artssouthern', 'artssprint', 'artsthe', 'artsuniversity', 'artswestchester', 'artwork', 'artym', 'arup', 'asc', 'ascites', 'ascp', 'asee', 'aseeking', 'aseptic', 'asheville', 'ashland', 'ashop', 'asia', 'asic', 'aside', 'asked', 'asp', 'aspect', 'aspects', 'aspire', 'aspirin', 'aspiring', 'asr', 'assay', 'assays', 'assemble', 'assembled', 'assembler', 'assemblies', 'assembling', 'assembly', 'assertive', 'assess', 'assessed', 'assessing', 'assessment', 'assessmento', 'assessments', 'assessmentwork', 'asset', 'assets', 'assign', 'assigned', 'assignedprogram', 'assigner', 'assignment', 'assignmento', 'assignments', 'assimilate', 'assist', 'assistance', 'assistant', 'assistantall', 'assistantberlin', 'assistantbeth', 'assistantcary', 'assistantcenter', 'assistantchaves', 'assistantdr', 'assistanteileen', 'assistanthobart', 'assistantkansas', 'assistantmood', 'assistantnorth', 'assistantohio', 'assistantoregon', 'assistantparo', 'assistantplant', 'assistants', 'assistantthe', 'assistantthomas', 'assistantus', 'assistantutah', 'assistantyale', 'assisted', 'assistedwith', 'assisting', 'assistive', 'assists', 'assoc', 'associate', 'associated', 'associatekarman', 'associateputnam', 'associates', 'associateschool', 'association', 'assorted', 'assortment', 'asst', 'assume', 'assumed', 'assumptions', 'assurance', 'assure', 'assured', 'assuring', 'ast', 'astc', 'astestate', 'asthma', 'astm', 'astrazeneca', 'astronomyboston', 'astrovirus', 'atcc', 'athens', 'atherosclerosis', 'athletes', 'athletic', 'athletics', 'atlantic', 'atm', 'atmel', 'atmosphere', 'atmospheric', 'atomic', 'atp', 'atr', 'atstexas', 'attached', 'attack', 'attain', 'attempt', 'attempted', 'attempts', 'attend', 'attendance', 'attended', 'attendees', 'attending', 'attends', 'attention', 'attentive', 'attenuation', 'attitude', 'attitudes', 'attorney', 'attorneys', 'attractants', 'attracting', 'attraction', 'attributes', 'attributing', 'atv', 'atwell', 'audience', 'audiences', 'audio', 'audit', 'audited', 'auditing', 'auditor', 'auditors', 'auditory', 'audits', 'aug', 'augmented', 'august', 'augusta', 'aurelia', 'ausaku', 'austin', 'australia', 'australiensis', 'author', 'authored', 'authoring', 'authorities', 'authority', 'authorizations', 'authorized', 'authoro', 'authors', 'autism', 'auto', 'autocad', 'autoclave', 'autoclaves', 'autoclaving', 'autocrine', 'automate', 'automated', 'automatic', 'automatically', 'automating', 'automation', 'automotive', 'autonomous', 'autoparts', 'autopsy', 'autosamplers', 'autotitrimeters', 'availability', 'available', 'average', 'averaging', 'avian', 'avidin', 'avifauna', 'avionics', 'award', 'awarddesigning', 'awarded', 'awardee', 'awardgiven', 'awardingthem', 'awardjune', 'awardmay', 'awards', 'awardsbeta', 'awardsdean', 'awardsieee', 'awardslisted', 'awardsscience', 'awardsthird', 'awareness', 'away', 'awic', 'awork', 'awpta', 'aws', 'awt', 'axes', 'axial', 'axis', 'azd', 'aznow', 'azornithology', 'baby', 'baccalaureate', 'bachelor', 'backflow', 'background', 'backgrounds', 'backlog', 'backpacking', 'backrubs', 'backside', 'bacteria', 'bacterial', 'bacteriologic', 'bacteriological', 'bacteriology', 'bacteriophage', 'bacterium', 'badge', 'badger', 'badges', 'bag', 'bait', 'bake', 'bakhtiari', 'bakhtiariqa', 'bakst', 'balance', 'balanced', 'balancelink', 'balds', 'ballistic', 'baltimore', 'bam', 'bamako', 'band', 'banded', 'banding', 'bandpasses', 'bands', 'bangalore', 'bangor', 'bank', 'banking', 'bankremote', 'banks', 'bannatyne', 'banquet', 'bar', 'barbara', 'barcode', 'bark', 'barn', 'barre', 'barrel', 'barrier', 'barriers', 'bartender', 'bartenderruby', 'bartending', 'bartlesville', 'base', 'based', 'baseline', 'basemap', 'bases', 'bash', 'basic', 'basics', 'basicîâ', 'basin', 'basins', 'basis', 'basking', 'bat', 'batch', 'bath', 'bathing', 'bathroom', 'baths', 'baton', 'bats', 'battlefield', 'baumann', 'bay', 'bayamoõ', 'bayes', 'bayesian', 'bbad', 'bbb', 'bbcwork', 'bbdedicated', 'bbf', 'bbhighly', 'bcbe', 'bcc', 'bccaa', 'bccf', 'bcf', 'bcie', 'bcmd', 'bdevelop', 'bdh', 'beach', 'beam', 'beans', 'bear', 'bearings', 'beautification', 'beaver', 'bebbcc', 'bec', 'beckman', 'bed', 'bedford', 'bedrock', 'beds', 'beech', 'beekeeping', 'beer', 'beerworks', 'beetle', 'beetles', 'bef', 'began', 'beginning', 'beginnings', 'behavior', 'behavioral', 'behaviorbiology', 'behaviors', 'behaviours', 'belgrade', 'believe', 'bell', 'bellmen', 'bellows', 'belonged', 'belowthe', 'belt', 'beltoctober', 'beltsville', 'bench', 'benchmark', 'benchmarks', 'benchmarkso', 'beneficiaries', 'benefit', 'benefits', 'benjamin', 'bennington', 'bent', 'benzene', 'benzodiazepines', 'beol', 'beowulf', 'beowulfhttp', 'beowulfnorwich', 'berkeley', 'berkley', 'berlin', 'bernstein', 'berry', 'best', 'bet', 'beta', 'beth', 'bethel', 'bethesda', 'bethlehem', 'better', 'bevel', 'beynnon', 'bho', 'bhutan', 'bhutantook', 'biannual', 'biannually', 'bid', 'bidding', 'big', 'bigblueîâ', 'bigger', 'bilateral', 'billable', 'billerica', 'billing', 'billion', 'bimonthly', 'bind', 'binding', 'bio', 'bioburden', 'biochem', 'biochemical', 'biochemist', 'biochemistry', 'biochip', 'bioconductor', 'biodiesel', 'biodiversity', 'bioesters', 'biofeedback', 'biogenesis', 'biohazardous', 'biohazards', 'bioinformatics', 'biol', 'biological', 'biologically', 'biologist', 'biologistiap', 'biologistport', 'biologists', 'biologistu', 'biologistusda', 'biology', 'biologybat', 'biologybrandeis', 'biologyharvard', 'biologyjames', 'biologymay', 'biologynorwich', 'biologysaint', 'biologyserver', 'biologyshandong', 'biologysouthern', 'biologyst', 'biologystate', 'biologythe', 'biologytrinity', 'biologywayne', 'biologyyale', 'biomarker', 'biomass', 'biomechanical', 'biomechanist', 'biomedcentral', 'biomedical', 'biometric', 'biomolecules', 'biomonitoring', 'bioone', 'biophotometer', 'biophys', 'biophysical', 'biophysics', 'biopsy', 'bioremediation', 'biorepository', 'bioretention', 'biosafety', 'bioscience', 'biosciences', 'biosolids', 'biostatistics', 'biosys', 'biotech', 'biotechnol', 'biotechnology', 'biotek', 'biotic', 'bioworks', 'bipolar', 'bipropellant', 'bird', 'birds', 'birkitt', 'birt', 'birth', 'bit', 'bites', 'bitten', 'biweekly', 'biz', 'black', 'blackboard', 'blacksburg', 'blading', 'blanket', 'blast', 'blaustein', 'bleeding', 'blend', 'blender', 'blepo', 'blight', 'blitz', 'blob', 'block', 'blocking', 'blocks', 'blog', 'blogs', 'blood', 'bloodmeal', 'bloomberg', 'blot', 'blots', 'blotting', 'blowers', 'bls', 'blsmay', 'blue', 'blueberries', 'blueîâ', 'bmc', 'bmcgenomics', 'bme', 'bms', 'board', 'boarded', 'boards', 'boat', 'boats', 'boback', 'boca', 'bod', 'bodies', 'body', 'boeing', 'bohm', 'bohmsenior', 'boise', 'boldoth', 'bolivia', 'boliviabetween', 'bolivian', 'bom', 'bomb', 'bond', 'bonds', 'bone', 'bonnie', 'bono', 'book', 'booked', 'bookeeping', 'booking', 'bookings', 'bookkeeping', 'books', 'bookseries', 'boost', 'booths', 'boots', 'bootstrap', 'border', 'borderline', 'borders', 'borne', 'boschnew', 'bosnia', 'boston', 'bosworth', 'botanical', 'botanist', 'botany', 'bottlenecks', 'bottling', 'boulder', 'boundaries', 'bourn', 'boutique', 'bovine', 'bow', 'box', 'boxcontacts', 'boxes', 'boy', 'boylan', 'boylanvice', 'boys', 'boz', 'bozeman', 'bpa', 'bpostdoctoral', 'brachial', 'brad', 'bradford', 'bradley', 'brady', 'bragg', 'brain', 'brainbow', 'brainmedia', 'brainstem', 'brainstorming', 'branch', 'branches', 'branching', 'brand', 'brands', 'brattleboro', 'brazil', 'brdu', 'brea', 'breach', 'breadth', 'break', 'breakdown', 'breakfast', 'breaking', 'breaks', 'breakup', 'breast', 'bred', 'breed', 'breeding', 'brenda', 'brett', 'breve', 'brew', 'brewer', 'brewery', 'brewing', 'briar', 'bridge', 'briefing', 'briefings', 'bright', 'brighter', 'brightfield', 'brighton', 'bring', 'bringing', 'bristol', 'britain', 'british', 'brittany', 'broad', 'broadcasting', 'broader', 'broadest', 'brochures', 'brody', 'broker', 'brokering', 'bromide', 'bronze', 'brook', 'brought', 'broughtartists', 'brown', 'brownfield', 'brownfields', 'browser', 'browsers', 'brucella', 'bruening', 'bruesewitz', 'brunswick', 'brustlin', 'bsl', 'bsponsored', 'budget', 'budgetary', 'budgeting', 'budgets', 'budish', 'buffalo', 'buffers', 'buffet', 'bug', 'bugs', 'bugzilla', 'build', 'builder', 'building', 'buildings', 'built', 'bulgaria', 'bulk', 'buncombe', 'bunkers', 'burbank', 'bureau', 'burkhard', 'burlington', 'burlingtoncore', 'burlingtonvt', 'burn', 'burned', 'burners', 'burns', 'burrows', 'burton', 'bus', 'busa', 'bush', 'business', 'businessand', 'businesses', 'bussercannon', 'busserthree', 'busy', 'buyer', 'buyers', 'bwork', 'byrd', 'byrne', 'bywendy', 'caae', 'cab', 'cabot', 'cac', 'cacertificate', 'caching', 'cad', 'caf', 'cafdb', 'cafeteria', 'cafeõ', 'caffe', 'cage', 'cages', 'cal', 'calais', 'calcium', 'calculate', 'calculated', 'calculating', 'calculation', 'calculations', 'calculator', 'calculus', 'caldwell', 'calendar', 'calendars', 'calex', 'calf', 'calibrated', 'calibrating', 'calibration', 'calibrations', 'calibre', 'california', 'californiaas', 'calkins', 'called', 'calling', 'calls', 'callscve', 'calmly', 'calorimetry', 'calves', 'cambio', 'cambridge', 'came', 'camera', 'cameras', 'cameroon', 'camp', 'campaign', 'campaigns', 'campsites', 'campton', 'campus', 'campuses', 'campwide', 'canada', 'cancellations', 'cancer', 'cancerous', 'cancers', 'candidate', 'candidates', 'cannabinoid', 'cannabinoids', 'canonical', 'canopy', 'canton', 'canyon', 'cap', 'capa', 'capabilities', 'capable', 'capably', 'capacitance', 'capacity', 'capecitabine', 'capillary', 'capital', 'capitalize', 'captivating', 'captive', 'capture', 'capturing', 'carbon', 'carbondale', 'carbonyl', 'carcasses', 'carcinoma', 'card', 'cardiac', 'cardio', 'cardiopulmonary', 'cardiovascular', 'cards', 'care', 'cared', 'career', 'careers', 'careervolunteer', 'carefully', 'cares', 'caribbean', 'caring', 'carlisle', 'carlo', 'carlos', 'carlsbad', 'carnegie', 'carnival', 'carolina', 'caroline', 'carolinensis', 'carotid', 'carpenter', 'carpenteryankee', 'carpentry', 'carried', 'carrier', 'carriers', 'carry', 'carrying', 'cars', 'cartilage', 'cartodb', 'cartography', 'carving', 'cas', 'cascade', 'cascades', 'case', 'cases', 'cash', 'cashier', 'cashiermendham', 'cashiers', 'caspase', 'cast', 'castilian', 'castleton', 'castro', 'castroenosburg', 'cat', 'catalog', 'catalogued', 'catalysis', 'catalysts', 'catalytic', 'categories', 'categorizing', 'category', 'catering', 'cateringall', 'catherine', 'catheter', 'cathinone', 'cathodic', 'catos', 'causative', 'cause', 'caused', 'causes', 'caving', 'cays', 'cbdwilling', 'cbeb', 'cbf', 'cbfc', 'cbir', 'cca', 'ccaaseeking', 'cce', 'ccf', 'ccrpc', 'ccs', 'cct', 'cctv', 'ccv', 'cda', 'cdc', 'cdd', 'cdna', 'cdto', 'ceasing', 'cee', 'cefms', 'celery', 'cell', 'cellist', 'cells', 'cellular', 'celpconsultant', 'cement', 'census', 'censuses', 'centaur', 'centennial', 'center', 'centerat', 'centered', 'centers', 'centos', 'central', 'centralvermont', 'centre', 'centricity', 'centrifugal', 'centrifugation', 'centrifuge', 'centrifuging', 'centro', 'centroamericano', 'century', 'ceo', 'ceramic', 'cerner', 'certificate', 'certificateart', 'certificates', 'certification', 'certifications', 'certified', 'certifiedlab', 'certifying', 'cervical', 'cfaba', 'cfba', 'cfd', 'cfe', 'cff', 'cfi', 'cfletcher', 'cfo', 'cfr', 'cfusting', 'cga', 'cgi', 'cgmp', 'chad', 'chagas', 'chahwan', 'chain', 'chains', 'chainsaw', 'chair', 'chaired', 'chairing', 'chairman', 'challenge', 'challenged', 'challenges', 'challenging', 'chamber', 'chambers', 'chameleon', 'champ', 'champion', 'championed', 'championships', 'champlain', 'chance', 'change', 'changeover', 'changes', 'changing', 'changzhou', 'channel', 'chapel', 'chapter', 'character', 'characteristic', 'characteristics', 'characterize', 'characterized', 'characterizing', 'characteriztion', 'charge', 'charged', 'charges', 'charles', 'charleston', 'charlotte', 'charlottesville', 'chart', 'charted', 'charter', 'chartered', 'charting', 'charts', 'chase', 'chases', 'chasse', 'chasseõ', 'chatham', 'chaube', 'check', 'checked', 'checking', 'checkpoint', 'checks', 'cheerful', 'chefaramark', 'chefs', 'chelated', 'chelsea', 'chem', 'chemdrawîâ', 'chemical', 'chemically', 'chemicals', 'chemist', 'chemistabbott', 'chemistblistex', 'chemistperrigo', 'chemistries', 'chemistry', 'chemistrybates', 'chemistrybutler', 'chemistryhttp', 'chemistrykansas', 'chemistrykeene', 'chemistryking', 'chemistrymay', 'chemistrypurdue', 'chemistrystate', 'chemistrythe', 'chemists', 'chemistself', 'chemokine', 'chemostat', 'chemotherapy', 'chemsketch', 'chemstation', 'chen', 'chennai', 'chest', 'chester', 'chestnut', 'chevron', 'chicago', 'chicken', 'chief', 'chiefs', 'chieftown', 'child', 'childhood', 'children', 'chile', 'chiller', 'chimborazo', 'china', 'chinabs', 'chinese', 'chip', 'chipotle', 'chips', 'chipset', 'chittenden', 'chloride', 'chlorinated', 'chlorination', 'chlorobiphenyl', 'chlorophyll', 'choe', 'choi', 'choice', 'choices', 'cholera', 'cholic', 'chondrichthyan', 'choose', 'choosing', 'chorale', 'chores', 'chosen', 'chris', 'christer', 'christina', 'christopher', 'chroma', 'chromatograph', 'chromatographed', 'chromatographs', 'chromatography', 'chromogenic', 'chronic', 'chuck', 'chuckles', 'chuquisaca', 'church', 'cia', 'ciat', 'cichanowski', 'cie', 'cimg', 'cinachyrella', 'cindy', 'cipt', 'circle', 'circles', 'circuit', 'circuitry', 'circulating', 'circulation', 'circumpolar', 'circumstances', 'cis', 'cisco', 'cited', 'citizen', 'citizens', 'citizenship', 'citrix', 'city', 'civil', 'cjay', 'claim', 'claims', 'claremont', 'clarify', 'class', 'classes', 'classical', 'classification', 'classifier', 'classify', 'classroom', 'classrooms', 'classroomuniv', 'clauson', 'clay', 'clays', 'clean', 'cleaned', 'cleaning', 'cleanliness', 'cleans', 'cleansers', 'cleanup', 'clear', 'cleared', 'clearing', 'clearly', 'clearslide', 'clemson', 'clergy', 'clerical', 'clerks', 'cleveland', 'clia', 'client', 'clientele', 'clients', 'climate', 'climates', 'climatic', 'climatologic', 'climb', 'climbing', 'clinial', 'clinic', 'clinical', 'clinically', 'clinician', 'clinics', 'clinton', 'clipper', 'clipping', 'clips', 'clone', 'cloned', 'clones', 'cloning', 'close', 'closed', 'closely', 'closes', 'closing', 'closure', 'cloths', 'cloud', 'clover', 'club', 'clubs', 'clubthe', 'cluster', 'clustering', 'clustero', 'clusters', 'cmburlington', 'cmead', 'cmms', 'cmos', 'cms', 'cnc', 'cns', 'coach', 'coached', 'coaching', 'coachsau', 'coachusa', 'coachwindham', 'coag', 'coagulation', 'coast', 'coastal', 'coating', 'cobas', 'cobra', 'cobre', 'cochlea', 'cochlear', 'cockaded', 'cocobolo', 'cod', 'code', 'codec', 'codecteam', 'coded', 'coder', 'codes', 'codeveloping', 'codewarrior', 'coding', 'coffee', 'cogeneration', 'cognex', 'cognitive', 'cognitiveworks', 'cognizant', 'cogntivewx', 'cohesive', 'cohort', 'cohorts', 'colby', 'colchester', 'cold', 'coli', 'coliform', 'coliforms', 'colilert', 'collaborate', 'collaborated', 'collaborates', 'collaborating', 'collaboration', 'collaborations', 'collaborative', 'collaboratively', 'collar', 'colleagues', 'collect', 'collected', 'collecting', 'collection', 'collections', 'collector', 'collectors', 'college', 'collegebomoseen', 'collegegreen', 'collegelinks', 'colleges', 'collegeville', 'collegiate', 'collins', 'colombia', 'colonies', 'colony', 'color', 'colorado', 'coloration', 'colored', 'columbia', 'columbus', 'column', 'com', 'comadditional', 'comasheville', 'combination', 'combined', 'combustion', 'come', 'comengineer', 'comexecutive', 'comfort', 'comfortable', 'comibm', 'coming', 'command', 'commanded', 'commands', 'commaster', 'commemorate', 'commencing', 'comment', 'commenting', 'comments', 'commerce', 'commercial', 'commission', 'commissioner', 'commissionikb', 'commissioning', 'commissions', 'commitment', 'commitments', 'committed', 'committee', 'committees', 'common', 'commonly', 'commun', 'communicate', 'communicated', 'communicating', 'communication', 'communications', 'communicationso', 'communicator', 'communities', 'community', 'comp', 'compact', 'compaction', 'companies', 'company', 'companysouth', 'companytemp', 'companywide', 'comparative', 'compared', 'comparing', 'comparison', 'compass', 'compatibility', 'compelling', 'compendium', 'compensated', 'compensation', 'competed', 'competence', 'competencies', 'competency', 'competent', 'competing', 'competition', 'competitions', 'competitive', 'compilation', 'compile', 'compiled', 'compiler', 'compiling', 'complaints', 'complement', 'complementary', 'complete', 'completed', 'completely', 'completeness', 'completing', 'completion', 'completive', 'complex', 'complexes', 'complexities', 'compliance', 'compliant', 'complications', 'comply', 'complying', 'component', 'components', 'composed', 'composing', 'composite', 'composition', 'compostinglime', 'compound', 'compounded', 'compounder', 'compounds', 'comprehensibly', 'comprehension', 'comprehensive', 'compress', 'compressed', 'compression', 'compressor', 'compressors', 'comprised', 'comptroller', 'compustat', 'computation', 'computational', 'computationally', 'compute', 'computerized', 'computers', 'computing', 'computingapril', 'comsandhill', 'comunity', 'concave', 'concealed', 'conceived', 'concentration', 'concentrations', 'concept', 'concepts', 'conceptso', 'conceptual', 'conceptualized', 'concern', 'concerned', 'concerning', 'concerns', 'concert', 'concerts', 'concise', 'concisely', 'conclusion', 'conclusionpcr', 'conclusionthe', 'concord', 'concrete', 'condition', 'conditioning', 'conditions', 'condor', 'conduct', 'conducted', 'conducting', 'conductivity', 'conductor', 'conducts', 'cone', 'conference', 'conferences', 'confidence', 'confidential', 'confidentiality', 'config', 'configuration', 'configurations', 'configure', 'configured', 'configuring', 'confined', 'confirm', 'confirmations', 'confirmed', 'confirming', 'confiscating', 'conflict', 'confluence', 'confocal', 'conformance', 'conforming', 'confronted', 'confused', 'congaree', 'congenic', 'congress', 'conicit', 'conjugation', 'conjunction', 'conn', 'connect', 'connecticut', 'connecting', 'connection', 'connections', 'connects', 'connolly', 'conscientious', 'consecutive', 'consensus', 'consensuso', 'consent', 'consents', 'conservation', 'conserve', 'consider', 'consideration', 'considered', 'considering', 'considers', 'consisted', 'consistency', 'consistent', 'consistently', 'consisting', 'consists', 'console', 'consoles', 'consolidated', 'consolidation', 'constitutive', 'constraints', 'construct', 'constructed', 'constructing', 'construction', 'consultancy', 'consultant', 'consultantemcon', 'consultantnasa', 'consultants', 'consultantself', 'consultantus', 'consultantusaa', 'consultation', 'consultations', 'consulted', 'consulting', 'consumable', 'consumed', 'consumer', 'consumers', 'consumption', 'contact', 'contacted', 'contacts', 'contained', 'container', 'containers', 'containing', 'containment', 'contaminants', 'contaminated', 'contamination', 'conte', 'contemporary', 'content', 'contents', 'contest', 'contested', 'context', 'contextproject', 'contexts', 'contingency', 'continuation', 'continue', 'continued', 'continues', 'continuing', 'continuous', 'continuously', 'contour', 'contract', 'contracted', 'contracting', 'contractmoscow', 'contractor', 'contractorcora', 'contractors', 'contracts', 'contrast', 'contribute', 'contributed', 'contributing', 'contribution', 'contributions', 'contributor', 'contributors', 'control', 'controlled', 'controller', 'controllers', 'controlling', 'controls', 'controlxi', 'controversial', 'convention', 'conventional', 'conventions', 'convergence', 'converse', 'conversion', 'convert', 'converting', 'convey', 'conveyors', 'conway', 'conwayseeking', 'cook', 'cookcity', 'cookcreated', 'cooke', 'cooking', 'cooknew', 'cooks', 'cool', 'cooling', 'cooper', 'cooperation', 'cooperative', 'coopetarraz', 'coordinate', 'coordinated', 'coordinates', 'coordinating', 'coordination', 'coordinator', 'coordinatorleo', 'coordinators', 'coordinatorsd', 'coordinatorst', 'coorg', 'copeland', 'copper', 'coprincipal', 'coral', 'corals', 'cordella', 'cordova', 'core', 'coredfn', 'coregulation', 'corepressor', 'coreuniversity', 'coris', 'coristafebruary', 'corn', 'cornel', 'cornell', 'corning', 'cornish', 'coronal', 'corp', 'corporate', 'corporation', 'corporations', 'corps', 'corrals', 'correct', 'corrected', 'correction', 'correctional', 'corrections', 'corrective', 'correlated', 'correlation', 'correlations', 'correspond', 'correspondence', 'corresponding', 'corridor', 'corrpro', 'cortical', 'cortunix', 'corvallis', 'cosmetic', 'cost', 'costa', 'costadrica', 'costing', 'costs', 'cotto', 'coturnix', 'coulter', 'council', 'counsel', 'counseled', 'counseling', 'counselor', 'counselors', 'counselprivate', 'counsels', 'count', 'counter', 'counteracts', 'countermeasure', 'counterparts', 'counters', 'counting', 'countries', 'country', 'counts', 'county', 'coupled', 'coupling', 'courage', 'courier', 'course', 'courseresearch', 'courses', 'coursework', 'courteous', 'courtroom', 'covalent', 'cover', 'coverage', 'covered', 'covering', 'cow', 'cowbird', 'cowbirds', 'coworkers', 'cpe', 'cpeo', 'cpr', 'cprapril', 'cpt', 'crab', 'crack', 'cracks', 'craft', 'crafted', 'crafting', 'craftsbury', 'craftsman', 'craftsmanship', 'craftsmen', 'crane', 'crash', 'crassa', 'crater', 'craters', 'crea', 'cream', 'creams', 'create', 'created', 'creating', 'creatinine', 'creation', 'creationdata', 'creative', 'creativity', 'creator', 'credential', 'credentialing', 'credentials', 'credibility', 'credible', 'credit', 'creek', 'crest', 'crew', 'crews', 'crises', 'crisesin', 'crispr', 'criteria', 'critical', 'critically', 'criticism', 'critiques', 'critiquing', 'crkl', 'crm', 'crna', 'crop', 'crops', 'cros', 'cross', 'crrel', 'crrt', 'cruise', 'cruising', 'crushes', 'crust', 'cruzi', 'cryo', 'cryophysics', 'cryptography', 'cryptosporidium', 'crystal', 'crystals', 'csa', 'cseeking', 'css', 'csssenior', 'csssoftware', 'ctcertification', 'cto', 'ctoinformation', 'ctt', 'cukan', 'cull', 'culling', 'culminated', 'culminating', 'culmination', 'cultivated', 'cultivating', 'cultivation', 'cultural', 'culture', 'cultured', 'cultures', 'culturing', 'culvert', 'culverts', 'cup', 'curator', 'curled', 'curling', 'currency', 'current', 'currently', 'currentsensing', 'curricular', 'curriculum', 'curve', 'curves', 'custody', 'custom', 'customcommands', 'customer', 'customers', 'customized', 'customizing', 'customs', 'cutting', 'cve', 'cvs', 'cwe', 'cwilling', 'cwork', 'cxcr', 'cyanamid', 'cyanide', 'cyber', 'cyberlab', 'cybersecurity', 'cycle', 'cycles', 'cyclic', 'cycling', 'cykon', 'cykonascp', 'cylinders', 'cynomolgus', 'cynthia', 'cysteine', 'cytokine', 'cytokines', 'cytometer', 'cytometry', 'cytometryelisa', 'cytoplasmic', 'cytotoxicity', 'cytoxicity', 'dabruese', 'dactyl', 'dad', 'daffodil', 'daily', 'dairy', 'daiwa', 'dakota', 'dakotas', 'dallas', 'dalt', 'dalvik', 'damage', 'damaged', 'damagegrowth', 'damages', 'dana', 'dance', 'dances', 'dancing', 'dania', 'daniel', 'dao', 'daphnia', 'dark', 'darkfield', 'darling', 'dart', 'dartmouse', 'dartmouth', 'dasatinib', 'dashboard', 'dashboards', 'data', 'database', 'databases', 'datacollection', 'dataindependent', 'datalab', 'dataloggers', 'datascience', 'datascientist', 'datasets', 'date', 'dates', 'david', 'davidson', 'davidsono', 'davis', 'dawley', 'dawn', 'day', 'daybackpacking', 'daylight', 'days', 'dba', 'dbase', 'dbd', 'dbms', 'dca', 'dcc', 'dcskillslabview', 'dct', 'dcwilling', 'ddb', 'ddfd', 'deaconess', 'dead', 'deadlines', 'deadlinesgis', 'deadlinessummer', 'deal', 'dealers', 'dealing', 'dean', 'deanlindsey', 'deans', 'death', 'debian', 'deborah', 'debris', 'debt', 'debug', 'debugging', 'dec', 'deca', 'decade', 'decades', 'december', 'deciding', 'decipher', 'decision', 'decisions', 'decisive', 'deck', 'declarations', 'declineavian', 'decontaminate', 'deconvolution', 'decorated', 'decorum', 'decrease', 'dedicated', 'deductions', 'deep', 'deer', 'deering', 'defeat', 'defect', 'defects', 'defending', 'defense', 'define', 'defined', 'defining', 'definition', 'deflated', 'deflectometer', 'defuse', 'degenerative', 'degradation', 'degree', 'degrees', 'del', 'delaware', 'delay', 'delayed', 'delayering', 'delays', 'delegate', 'delegated', 'delegates', 'delegation', 'delhi', 'deliberations', 'deliberative', 'delicate', 'delineated', 'delineating', 'delineation', 'delineations', 'deliver', 'deliverable', 'deliverables', 'delivered', 'deliveries', 'delivering', 'delivers', 'delivery', 'deliveryproduct', 'delmilagro', 'delta', 'demand', 'demanded', 'dementia', 'demeter', 'demo', 'democracyintern', 'democratic', 'demolition', 'demonstrate', 'demonstrated', 'demonstrates', 'demonstration', 'demonstrations', 'demospongiae', 'dempster', 'denaturation', 'denis', 'denise', 'denominations', 'densitometry', 'density', 'dental', 'dentistry', 'deodorants', 'department', 'departmental', 'departmentre', 'departments', 'depend', 'dependable', 'dependencies', 'dependent', 'depending', 'dependingon', 'deploy', 'deployed', 'deployment', 'deployments', 'deposition', 'depositions', 'deposits', 'depression', 'deprivation', 'dept', 'depth', 'depuration', 'deputy', 'derailment', 'derivatives', 'derived', 'desc', 'describes', 'describing', 'description', 'descriptions', 'descriptive', 'desert', 'design', 'designated', 'designation', 'designblueprint', 'designbusa', 'designed', 'designer', 'designergvh', 'designeribm', 'designers', 'designhampshire', 'designing', 'designkineticom', 'designs', 'desire', 'desired', 'desk', 'desktop', 'despite', 'destroy', 'destroyer', 'detached', 'detailed', 'detailing', 'details', 'detect', 'detected', 'detecting', 'detection', 'detectionneural', 'detector', 'determinants', 'determination', 'determinations', 'determine', 'determined', 'determines', 'determining', 'detracted', 'detroit', 'deutsche', 'devaux', 'develop', 'developed', 'developer', 'developeraquent', 'developerats', 'developercsl', 'developermerkle', 'developero', 'developerrolls', 'developers', 'developersun', 'developerthe', 'developerthird', 'developerusaa', 'developerusc', 'developerwipro', 'developing', 'development', 'developmental', 'developmentally', 'developments', 'develops', 'deviation', 'deviations', 'device', 'devices', 'devise', 'dfs', 'dhahran', 'dhs', 'diabetes', 'diagnose', 'diagnosed', 'diagnoses', 'diagnosing', 'diagnosis', 'diagnostic', 'diagnostico', 'diagnostics', 'diagrams', 'dialogs', 'dialogue', 'diapause', 'dic', 'dichotomous', 'dickinson', 'dictionaries', 'dictionary', 'die', 'died', 'diego', 'diem', 'diesel', 'dietary', 'differences', 'different', 'differential', 'differentials', 'differentiation', 'differing', 'differs', 'difficult', 'difficulties', 'diffraction', 'diffused', 'diffusers', 'diffusing', 'diffusion', 'dig', 'digestion', 'digestions', 'digital', 'digitally', 'digitize', 'digitized', 'dignity', 'digoxigenin', 'dijkstra', 'diligence', 'diligent', 'diluted', 'dilutions', 'dimension', 'dimensional', 'dimensioning', 'dimensions', 'dimidiata', 'dining', 'dinners', 'dionex', 'dioxin', 'diphtheria', 'diploma', 'diplomacy', 'diplomaenosburg', 'diplomageneral', 'diplomamelrose', 'diplomanorth', 'diplomatic', 'dipt', 'direct', 'directed', 'directing', 'direction', 'directions', 'directives', 'directly', 'director', 'directorate', 'directorboy', 'directorcamp', 'directorclassic', 'directors', 'directorvermont', 'directory', 'directv', 'dirichlet', 'disabilities', 'disability', 'disabled', 'disaster', 'discarded', 'discarding', 'discharge', 'disciplinary', 'discipline', 'disciplines', 'disclosed', 'disclosures', 'discounts', 'discover', 'discovered', 'discoverer', 'discoveries', 'discovering', 'discovery', 'discrepancy', 'discrete', 'discriminate', 'discriminating', 'discuss', 'discussed', 'discussing', 'discussion', 'discussions', 'disease', 'diseased', 'diseases', 'diseaseshttp', 'dish', 'dishwasher', 'dishwashing', 'disorder', 'disorders', 'disordersclinic', 'disparate', 'dispatch', 'dispatching', 'dispensed', 'dispensing', 'dispersal', 'dispersant', 'display', 'displayed', 'displaying', 'displayresearch', 'displays', 'disposal', 'disposing', 'disposition', 'dispositioned', 'disruption', 'disruptions', 'disruptive', 'dissected', 'dissection', 'dissections', 'disseminate', 'disseminated', 'disseminating', 'dissemination', 'dissertation', 'dissertations', 'dissolved', 'distance', 'distances', 'distant', 'distillate', 'distillation', 'distinct', 'distinction', 'distinctions', 'distinguish', 'distinguished', 'distortion', 'distressed', 'distributed', 'distributing', 'distribution', 'distributions', 'district', 'districts', 'districtstate', 'diurnally', 'dive', 'divemasters', 'divergent', 'diverse', 'diversified', 'diversions', 'diversity', 'dives', 'divestitures', 'divide', 'divided', 'diving', 'division', 'divisioncdc', 'divisionriver', 'divisionu', 'dlx', 'dmm', 'dmv', 'dmvs', 'dna', 'dnareal', 'dns', 'dnssupported', 'doc', 'docent', 'docker', 'docklight', 'dockside', 'docosahexaenoic', 'docs', 'doctor', 'doctoral', 'doctors', 'document', 'documentation', 'documented', 'documenting', 'documents', 'documentsput', 'dod', 'doe', 'dog', 'doghandling', 'dogs', 'dohmh', 'doi', 'dol', 'dollar', 'dollars', 'domain', 'domains', 'domestic', 'domestically', 'dominant', 'dominate', 'dominion', 'domino', 'donate', 'donated', 'donating', 'donation', 'donations', 'donor', 'donors', 'doppler', 'dorf', 'dormant', 'dormitories', 'dorsal', 'dorset', 'dos', 'dose', 'dosimetry', 'dosing', 'dot', 'dotpf', 'dots', 'douglas', 'download', 'dozen', 'dozend', 'dozens', 'dpn', 'dposition', 'dpw', 'dra', 'draft', 'drafted', 'drafting', 'drafts', 'drag', 'drags', 'drainage', 'dramatic', 'drawbacks', 'drawing', 'drawings', 'draws', 'dream', 'dreamliner', 'dreamweaver', 'dredging', 'dressing', 'drew', 'drill', 'drilling', 'drills', 'drink', 'drinking', 'drive', 'driven', 'driver', 'driverjunior', 'driverkingdom', 'drivers', 'driververmont', 'drives', 'driving', 'drone', 'droots', 'drop', 'drops', 'drove', 'drug', 'drugs', 'dry', 'dryer', 'drying', 'dsa', 'dsaresearch', 'dsc', 'dsp', 'dss', 'dsx', 'dto', 'dual', 'dubai', 'dudley', 'dumping', 'duration', 'durb', 'durham', 'duties', 'dutiessenior', 'duty', 'duxbury', 'dwelling', 'dwork', 'dyer', 'dynamic', 'dynamics', 'dynamicskarman', 'dynamicsmaster', 'dyne', 'eaa', 'eagan', 'eager', 'eagle', 'eao', 'ear', 'earlier', 'early', 'earned', 'earnings', 'earth', 'earthenware', 'earthwatch', 'earthworm', 'ease', 'easily', 'east', 'eastern', 'easterntropical', 'easy', 'eawork', 'eberl', 'ebf', 'ebfe', 'ebrd', 'ebsd', 'ecawork', 'ecb', 'ececfccreative', 'ecf', 'eclipse', 'eco', 'ecoagriculture', 'ecohealth', 'ecological', 'ecologistjeff', 'ecology', 'ecologypurdue', 'ecologyschool', 'ecologyyale', 'econ', 'economic', 'economics', 'economicsstate', 'economies', 'economist', 'economy', 'economyadjunct', 'ecostudies', 'ecosystem', 'ecosystems', 'ecotoxicology', 'ecs', 'ectoderm', 'edge', 'edges', 'edgewater', 'edi', 'edible', 'edit', 'edited', 'editing', 'edition', 'editor', 'editoracademic', 'editorial', 'editorids', 'editornorwich', 'editorself', 'editorworld', 'edits', 'eds', 'edu', 'educate', 'educated', 'educating', 'education', 'educationa', 'educational', 'educationb', 'educationba', 'educationbs', 'educationdoctor', 'educationm', 'educationmarine', 'educationmaster', 'educationms', 'educationph', 'educationphd', 'educationpost', 'educationst', 'educator', 'edwards', 'edwork', 'edxa', 'eed', 'eesc', 'efb', 'efe', 'effect', 'effected', 'effective', 'effectively', 'effectiveness', 'effector', 'effects', 'efficacy', 'efficiencies', 'efficiency', 'efficient', 'efficiently', 'effluents', 'effort', 'efforts', 'eform', 'egetation', 'egfr', 'egg', 'eggs', 'ehr', 'ehrlich', 'ehs', 'eia', 'eid', 'eigenface', 'eighth', 'eileen', 'eisenberg', 'eisenia', 'ejb', 'ejbs', 'ejbv', 'ejoramiento', 'ekg', 'eksigent', 'elasmobranch', 'elastic', 'elderly', 'elecronics', 'elected', 'election', 'electric', 'electrical', 'electro', 'electrode', 'electron', 'electronic', 'electronically', 'electronics', 'electrophoresis', 'electroshocking', 'electrostatic', 'elem', 'element', 'elementary', 'elements', 'elevation', 'eligibility', 'eliminate', 'eliminated', 'eliminating', 'elimination', 'elisa', 'elisas', 'elite', 'elizabeth', 'ellen', 'ellis', 'elmer', 'elmo', 'elucidate', 'elucidation', 'elzohairy', 'ema', 'email', 'emails', 'embankment', 'embedded', 'embedding', 'embodiment', 'embroidery', 'embryo', 'embryonal', 'embryonic', 'emergence', 'emergency', 'emerging', 'emeryville', 'emile', 'emissions', 'emitted', 'emotion', 'emphasis', 'emphasized', 'emphasizing', 'empire', 'empirical', 'employability', 'employed', 'employee', 'employees', 'employeestone', 'employers', 'employerwork', 'employment', 'employs', 'emr', 'emulsions', 'ena', 'enable', 'enabled', 'enablement', 'enables', 'enabling', 'enclosure', 'enclosures', 'encoder', 'encoding', 'encompassing', 'encounter', 'encounters', 'encourage', 'encouraged', 'encryption', 'encyclopedia', 'end', 'endangered', 'endeavor', 'endeavors', 'ended', 'endemic', 'endocrine', 'endocrinology', 'endogenous', 'endorsed', 'endorsement', 'endosomal', 'endothelial', 'endotoxin', 'energetic', 'energies', 'energy', 'energy_', 'enforce', 'enforcement', 'enforcing', 'engage', 'engaged', 'engagement', 'engagements', 'engages', 'engine', 'engineeering', 'engineer', 'engineeralburgh', 'engineerapplied', 'engineerbio', 'engineerbiotek', 'engineercareer', 'engineerchroma', 'engineerctg', 'engineered', 'engineerfairfax', 'engineerford', 'engineerfujitsu', 'engineerge', 'engineergeneral', 'engineergw', 'engineerhoyle', 'engineeribm', 'engineering', 'engineeringthe', 'engineeringxi', 'engineerjuly', 'engineermilton', 'engineernanyou', 'engineernortech', 'engineerntrepid', 'engineerplanned', 'engineerrehau', 'engineers', 'engineersamsung', 'engineerstate', 'engineerthe', 'engineerusc', 'engineervarious', 'engineervintner', 'engineinfosys', 'engines', 'england', 'englandgreens', 'english', 'englishhigh', 'enhance', 'enhanced', 'enhancement', 'enhancements', 'enhancing', 'enjoy', 'enjoyment', 'enjoys', 'enjoyworking', 'enology', 'enord', 'enosburg', 'enriched', 'enrichment', 'enrolled', 'enrolling', 'enrollment', 'enrollments', 'ensemble', 'ensr', 'ensure', 'ensured', 'ensuring', 'entailed', 'ental', 'enter', 'entered', 'entering', 'enterprise', 'enterprises', 'entertain', 'entertained', 'entertainers', 'enthusiasm', 'enthusiastic', 'entire', 'entities', 'entitled', 'entitlement', 'entity', 'entomological', 'entomology', 'entrepreneur', 'entrepreneurial', 'entrez', 'entropy', 'entrusted', 'entry', 'entrydata', 'entryfield', 'entryindustrial', 'entryjp', 'enumerate', 'enumeration', 'enumerator', 'envi', 'environm', 'environment', 'environmental', 'environmentally', 'environmento', 'environments', 'envriomental', 'enzymatic', 'enzyme', 'enzymes', 'enzymology', 'eoae', 'eod', 'eop', 'epa', 'epi', 'epic', 'epidemiology', 'epileptic', 'episode', 'epitaxial', 'epithelial', 'epoxidation', 'epri', 'epscor', 'epsilon', 'epson', 'ept', 'equal', 'equation', 'equations', 'equine', 'equipment', 'equipmentthat', 'equipped', 'equivalent', 'eraoctober', 'erbitux', 'erdas', 'erdc', 'erennial', 'eric', 'erie', 'erik', 'erika', 'erinacea', 'erk', 'ernesto', 'erosion', 'erp', 'errands', 'errol', 'error', 'errors', 'ertem', 'erythreae', 'erythromycin', 'erô', 'erô_', 'escalated', 'escalation', 'esch', 'escherichia', 'ese', 'esearch', 'esi', 'esol', 'esp', 'especially', 'esri', 'essay', 'essential', 'essex', 'est', 'establish', 'established', 'establishing', 'establishment', 'establishments', 'esther', 'estimate', 'estimated', 'estimates', 'estimatics', 'estimating', 'estimation', 'estimator', 'estimators', 'estradiol', 'estrogenic', 'estuary', 'eszterhas', 'etch', 'etching', 'ethernet', 'ethic', 'ethical', 'ethics', 'ethidium', 'etiology', 'etiquette', 'etl', 'euclid', 'eugene', 'europe', 'european', 'evacuation', 'evaluate', 'evaluated', 'evaluating', 'evaluation', 'evaluations', 'evan', 'evance', 'evangelism', 'evangelizing', 'evanston', 'evening', 'evenings', 'event', 'events', 'eventually', 'everbank', 'everyday', 'evidence', 'evidentiary', 'evisiting', 'evoked', 'evola', 'evolution', 'evolutionary', 'evolved', 'evolving', 'ewing', 'ework', 'exam', 'examination', 'examinations', 'examine', 'examined', 'examining', 'example', 'examples', 'exams', 'excavation', 'exceed', 'exceeded', 'exceeding', 'excel', 'excellab', 'excelled', 'excellence', 'excellent', 'excelîâ', 'exception', 'exceptional', 'exceptionally', 'exceptions', 'excess', 'exchange', 'excited', 'exciting', 'exclusion', 'exclusive', 'excursions', 'executable', 'execute', 'executed', 'executing', 'execution', 'executive', 'executives', 'executiveso', 'exercise', 'exercises', 'exfiltration', 'exhibit', 'exhibited', 'exhibits', 'exist', 'existing', 'exists', 'exit', 'expand', 'expanded', 'expanding', 'expansion', 'expansions', 'expectation', 'expectations', 'expected', 'expedite', 'expedited', 'expediting', 'expedition', 'expense', 'expenses', 'experience', 'experienceall', 'experiencecity', 'experiencecook', 'experienced', 'experiencedata', 'experiencegis', 'experiencehead', 'experiencehuman', 'experiencelead', 'experiencelts', 'experiencemicro', 'experienceqa', 'experiencern', 'experiences', 'experiencesales', 'experiencesoul', 'experiencesport', 'experiencestaff', 'experiencetem', 'experiencetitle', 'experiencetown', 'experiencewater', 'experiencework', 'experienceyouth', 'experiencing', 'experiential', 'experiment', 'experimental', 'experiments', 'expert', 'expertise', 'experts', 'expertso', 'expires', 'explain', 'explained', 'explaining', 'explains', 'exploit', 'exploits', 'exploration', 'exploratory', 'explore', 'explored', 'exploring', 'explosive', 'explosives', 'expos', 'exposed', 'exposition', 'exposure', 'exposures', 'express', 'expressed', 'expression', 'ext', 'extend', 'extended', 'extending', 'extends', 'extension', 'extensions', 'extensive', 'extensivelarge', 'extensively', 'extent', 'extents', 'exterminated', 'external', 'externally', 'extinction', 'extra', 'extract', 'extracted', 'extraction', 'extractions', 'extrapolate', 'extreme', 'extremely', 'extremes', 'extremophiles', 'extruded', 'extrusion', 'extrusions', 'exuma', 'eye', 'eyed', 'eyemed', 'faa', 'faad', 'fab', 'fabric', 'fabricated', 'fabrication', 'fabricator', 'fac', 'face', 'facebook', 'facets', 'facial', 'facilitate', 'facilitated', 'facilitating', 'facilitation', 'facilitator', 'facilities', 'facilitiespre', 'facility', 'facilitymanaged', 'facing', 'facs', 'facscanto', 'fact', 'factor', 'factors', 'factory', 'facts', 'faculty', 'facultynew', 'facultythe', 'faeb', 'fahc', 'faildata', 'failing', 'failover', 'failure', 'failures', 'fair', 'fairapril', 'fairbanks', 'fairfax', 'fairfield', 'fairlee', 'fairly', 'fairs', 'falco', 'fall', 'falling', 'fallopian', 'falls', 'familial', 'familiar', 'familiarity', 'familiarization', 'familiarizing', 'families', 'family', 'famous', 'fan', 'fans', 'fantastic', 'far', 'farber', 'farm', 'farmer', 'farmernortheast', 'farmers', 'farming', 'farms', 'fashion', 'fast', 'fastener', 'fasteners', 'faster', 'fastethernet', 'fasting', 'fat', 'father', 'fathom', 'fatigue', 'fatty', 'fault', 'faults', 'faulty', 'fauna', 'fava', 'favorova', 'fawork', 'fax', 'faxing', 'fazzino', 'fba', 'fbb', 'fbf', 'fcdb', 'fci', 'fcwilling', 'fda', 'fdcc', 'fdli', 'fdwilling', 'fea', 'fears', 'feasibility', 'feasible', 'feature', 'featured', 'features', 'featuring', 'feb', 'february', 'feburary', 'fecal', 'feces', 'fed', 'federal', 'federally', 'fedora', 'feed', 'feedback', 'feedbacko', 'feeding', 'feeds', 'feedstock', 'feel', 'fees', 'feet', 'fefwork', 'felicity', 'fellow', 'fellowecohealth', 'fellownorwich', 'fellows', 'fellowship', 'fellowtulane', 'fellowvt', 'fellowyale', 'fema', 'female', 'females', 'fence', 'fencing', 'ferc', 'fermentation', 'fernie', 'ferning', 'ferrocene', 'ferruginous', 'fertility', 'fertilizer', 'fertilizers', 'fertilizing', 'festival', 'festivals', 'fetal', 'fetida', 'ffb', 'ffrdc', 'fft', 'fhwa', 'fiber', 'fibers', 'fibroblast', 'fibrosis', 'fid', 'field', 'fielded', 'fieldnovember', 'fields', 'fieldtrips', 'fieldwork', 'fifteenhundred', 'fifth', 'fight', 'fighter', 'figures', 'figuring', 'file', 'filed', 'files', 'filesystem', 'filing', 'filings', 'filling', 'film', 'filmadditional', 'filter', 'filtered', 'filtering', 'filtero', 'filters', 'filtration', 'final', 'finalist', 'finalization', 'finalized', 'finals', 'finance', 'financial', 'financiers', 'financing', 'finding', 'findings', 'fine', 'finfish', 'finger', 'fingerprints', 'finish', 'finished', 'finishing', 'fink', 'finkdata', 'finley', 'finnigan', 'fired', 'firefighter', 'firefox', 'firewalls', 'firewallsweb', 'fireworks', 'firing', 'firm', 'firmkineticom', 'firmness', 'firms', 'fischer', 'fish', 'fisher', 'fisheries', 'fisheshttp', 'fishing', 'fishingrods', 'fishkill', 'fit', 'fitting', 'fiveo', 'fix', 'fixation', 'fixed', 'fixes', 'fixing', 'fixture', 'flagstaff', 'flame', 'flash', 'flat', 'flavors', 'fledglings', 'fleet', 'flesh', 'fletcher', 'flexibility', 'flexible', 'flicker', 'flight', 'flights', 'floating', 'flooding', 'floodplains', 'floor', 'floorartist', 'floorcumberland', 'flora', 'florida', 'flow', 'flower', 'flowjoamt', 'flows', 'flsouth', 'flu', 'fluency', 'fluent', 'fluid', 'fluids', 'fluke', 'fluorescence', 'fluorescent', 'fluorescently', 'flux', 'flx', 'fly', 'fmea', 'fmri', 'fnp', 'focal', 'focus', 'focused', 'focuses', 'focusing', 'foe', 'foil', 'folding', 'foliosissimum', 'folk', 'follow', 'followed', 'following', 'food', 'foods', 'foot', 'football', 'footed', 'footprint', 'footwear', 'foraging', 'force', 'forces', 'forecast', 'forecasted', 'forecasting', 'foreign', 'forensic', 'forest', 'forestal', 'forester', 'forestry', 'forests', 'forged', 'forklift', 'form', 'forma', 'formal', 'formaldehyde', 'format', 'formation', 'formatting', 'formed', 'forming', 'forms', 'formula', 'formulary', 'formulas', 'formulate', 'formulated', 'formulation', 'forr', 'forrest', 'forrester', 'forrests', 'fort', 'forth', 'fortran', 'fortune', 'forum', 'forward', 'forwarded', 'foster', 'fostering', 'foundation', 'foundations', 'founded', 'founder', 'founding', 'fourieranalysis', 'fourth', 'fpga', 'fqhc', 'fra', 'fracture', 'fragmentation', 'fragrance', 'fragrances', 'frame', 'frames', 'framework', 'frameworko', 'frameworks', 'frameworksenior', 'framingham', 'franchise', 'francisco', 'frank', 'franklin', 'free', 'freehand', 'freelance', 'freemantle', 'freesurfer', 'freeze', 'freezer', 'freezing', 'freight', 'french', 'frenchharvard', 'frequency', 'frequent', 'frequentist', 'frequently', 'fresh', 'fresheners', 'friend', 'friendly', 'frisbie', 'frisbieasst', 'frisco', 'froebel', 'frolov', 'fromvortex', 'frontal', 'fronttech', 'frost', 'frozen', 'fruit', 'fruits', 'frustration', 'fry', 'fsl', 'ftes', 'ftir', 'fuel', 'fuels', 'fulcrumapp', 'fuld', 'fulfilled', 'fuller', 'fully', 'fulvestrant', 'function', 'functional', 'functionalities', 'functionality', 'functionally', 'functioned', 'functionhttp', 'functioning', 'functions', 'fund', 'fundamental', 'fundamentals', 'funded', 'funding', 'fundraiser', 'fundraising', 'funds', 'fungal', 'furnace', 'furnaces', 'furthertraining', 'fusion', 'fusting', 'future', 'fuze', 'fwd', 'fwork', 'gaathens', 'gabapentin', 'gabor', 'gad', 'gages', 'gaging', 'gagnon', 'gain', 'gained', 'gaining', 'gains', 'galaxy', 'gale', 'galgotias', 'gallant', 'gallantsenior', 'galleries', 'gallery', 'galleryfour', 'gallons', 'gamble', 'game', 'games', 'gaming', 'gamma', 'gantry', 'gap', 'garden', 'gardener', 'gardening', 'gardens', 'gardner', 'garments', 'garnered', 'gas', 'gaseous', 'gases', 'gasoline', 'gate', 'gates', 'gather', 'gathered', 'gathering', 'gauging', 'gaussian', 'gauthier', 'gave', 'gcc', 'gcet', 'gcp', 'gcse', 'gct', 'gear', 'gears', 'gearx', 'ged', 'geds', 'gel', 'gels', 'genbank', 'gender', 'gene', 'genechips', 'geneious', 'geneotyping', 'genepop', 'general', 'generalist', 'generalized', 'generate', 'generated', 'generating', 'generation', 'generational', 'generations', 'generator', 'generic', 'genes', 'genetic', 'genetically', 'genetics', 'geneticsclemson', 'geneva', 'genome', 'genomic', 'genomics', 'genotoxicity', 'genotype', 'genotyping', 'gensuite', 'gentleness', 'geochemistry', 'geoffrey', 'geographers', 'geographic', 'geographical', 'geography', 'geoinformation', 'geologic', 'geological', 'geologist', 'geologists', 'geologiststone', 'geology', 'geologyst', 'geologythe', 'geomarine', 'geophone', 'geophysical', 'geophysicist', 'geophysics', 'geoprobe', 'georeferenced', 'george', 'georgegallant', 'georges', 'georgetown', 'georgia', 'geoscience', 'geosciences', 'geospatial', 'geostatistics', 'geosynthetic', 'geosynthetics', 'geotools', 'geovanny', 'geovannyjobs', 'geriatric', 'german', 'germany', 'germination', 'germs', 'gero', 'gerontology', 'gerry', 'gestation', 'gga', 'ggt', 'giaccone', 'giant', 'giardia', 'giasson', 'gibbs', 'giesel', 'gift', 'gifted', 'gillnets', 'gingerbread', 'giordano', 'giorgio', 'giovanni', 'girl', 'girls', 'gis', 'git', 'given', 'giving', 'glance', 'gland', 'glass', 'glasses', 'glassware', 'glaze', 'glazes', 'gleaned', 'gleevec', 'glen', 'glendale', 'glenn', 'glens', 'glenside', 'glenwater', 'glew', 'glm', 'glmm', 'glmnet', 'global', 'globally', 'globe', 'gloucester', 'glp', 'glucose', 'glutamyl', 'glycan', 'glyco', 'glycoconj', 'glycoengineered', 'glycofi', 'glycofithetford', 'glycoproteins', 'glycosylation', 'gmail', 'gmavt', 'gmbh', 'gmed', 'gmm', 'gmp', 'gnatcatcher', 'gnatcatchers', 'gnrh', 'gnu', 'goal', 'goals', 'goalseducationb', 'goddard', 'godwin', 'goers', 'goffstown', 'going', 'gold', 'goldathletics', 'goldengate', 'golf', 'gollnick', 'goo', 'good', 'goods', 'goodssenior', 'google', 'googleîâ', 'gopher', 'gorham', 'got', 'gov', 'governance', 'governing', 'government', 'governmental', 'governor', 'gpa', 'gpmaw', 'gps', 'gpu', 'grade', 'graded', 'grader', 'graders', 'grades', 'gradient', 'gradients', 'grading', 'graduate', 'graduatestudent', 'graduating', 'graduation', 'gram', 'granados', 'grand', 'grandfather', 'grant', 'granted', 'grants', 'grantsdeveloped', 'granular', 'grapes', 'graph', 'graphic', 'graphical', 'graphically', 'graphics', 'graphing', 'graphpad', 'graphs', 'grasping', 'grass', 'grasscity', 'grassroots', 'gravimetric', 'grazing', 'grc', 'gre', 'great', 'greater', 'greatly', 'greece', 'green', 'greenbelt', 'greenfield', 'greenhouse', 'greenhouses', 'greenville', 'greenwich', 'greeting', 'gregoritsch', 'grew', 'grid', 'grief', 'grievances', 'grinding', 'grocery', 'grooming', 'gross', 'ground', 'grounds', 'groundwater', 'groundwork', 'groundworks', 'group', 'groupberlex', 'groups', 'groupsamerican', 'groupseptember', 'groupsgreen', 'groupsindoor', 'groupskills', 'grove', 'grow', 'growing', 'grown', 'growth', 'growthresearch', 'gruppe', 'gsa', 'gst', 'gta', 'gtpases', 'guanacaste', 'guang', 'guard', 'guarentee', 'guatemala', 'guatemalan', 'guest', 'guests', 'guestscontact', 'gui', 'guidance', 'guide', 'guidearbortrek', 'guidebook', 'guided', 'guidelines', 'guides', 'guiding', 'guild', 'guinea', 'guis', 'guitar', 'gulf', 'gull', 'gun', 'guns', 'gurion', 'gut', 'guy', 'gym', 'gyms', 'gynecologic', 'gynecological', 'gynecology', 'haar', 'habitat', 'habitats', 'habits', 'haccp', 'hadley', 'hadoop', 'hadprimary', 'haemonetics', 'haiku', 'hailey', 'hale', 'haleigh', 'haleresearch', 'half', 'halfman', 'halibut', 'hall', 'halls', 'halogen', 'hamilton', 'hammond', 'hampers', 'hampshire', 'hampton', 'hand', 'handbook', 'handheld', 'handle', 'handled', 'handleproblems', 'handling', 'handouts', 'handpicked', 'handprint', 'hands', 'handyman', 'hanes', 'hangen', 'hanger', 'hanko', 'hanover', 'happenings', 'happiness', 'happinesstech', 'happy', 'happycustomers', 'harajr', 'harassment', 'harbors', 'hard', 'hardening', 'harding', 'hardness', 'hardware', 'hardwick', 'hardworking', 'harmon', 'harmony', 'harnesses', 'harrington', 'harrisonburg', 'harsh', 'hart', 'hartford', 'hartmann', 'hartnett', 'harvard', 'harvest', 'harwood', 'hat', 'haulers', 'hawk', 'haying', 'hayward', 'hazard', 'hazardous', 'hazards', 'hazcom', 'hazmat', 'hazmats', 'hazwoper', 'hbcd', 'hboc', 'hcpcs', 'head', 'headache', 'headcount', 'headed', 'heads', 'health', 'healthcare', 'healthjanuary', 'healthjohnson', 'healthy', 'heard', 'hearing', 'hearings', 'heart', 'heat', 'heath', 'heating', 'heavy', 'hectare', 'hedeman', 'hedging', 'heel', 'height', 'held', 'helena', 'helene', 'helicopter', 'helicopters', 'helifanplus', 'helmets', 'help', 'helpdesk', 'helped', 'helpedmentor', 'helper', 'helping', 'hemataphagous', 'hematology', 'hemiptera', 'hemmocult', 'hennessey', 'henry', 'hens', 'heor', 'hepatitis', 'hepatology', 'herbarium', 'herbicide', 'hercules', 'herd', 'heritage', 'heroes', 'herpetological', 'herzogenrath', 'hess', 'heterologous', 'heterotrophic', 'hhei', 'hibernate', 'hidden', 'hierarchical', 'high', 'highdimension', 'higher', 'highest', 'highland', 'highlight', 'highlighting', 'highlights', 'highly', 'highlyregarded', 'highway', 'highways', 'hikes', 'hiking', 'hilic', 'hill', 'hillsboro', 'hillsborough', 'hinesburg', 'hipaa', 'hippocampus', 'hire', 'hired', 'hires', 'hiring', 'hirise', 'histochemical', 'histogram', 'histological', 'histology', 'histopathology', 'historical', 'history', 'historyharvard', 'hitchcock', 'hiv', 'hixon', 'hjewett', 'hmm', 'hobart', 'hobo', 'hockey', 'holden', 'holder', 'holding', 'holdings', 'hole', 'holiday', 'holidays', 'hollow', 'holyoke', 'home', 'homeland', 'homeless', 'homeowners', 'homes', 'homesteads', 'homesvice', 'homework', 'homeworks', 'homogenate', 'honda', 'hone', 'honed', 'honest', 'honesty', 'honor', 'honorable', 'honoraria', 'honors', 'hope', 'hopes', 'hopewell', 'hoping', 'hopkins', 'horizontally', 'hormonal', 'hormone', 'horse', 'horseback', 'horses', 'horseshoe', 'hort', 'hortclemson', 'hospital', 'hospitality', 'hospitalrutland', 'hospitals', 'host', 'hostage', 'hosted', 'hosting', 'hostnorth', 'hosts', 'hot', 'hotdog', 'hotel', 'hotels', 'hotmail', 'hotspots', 'hour', 'hourly', 'hours', 'hourtwice', 'house', 'housed', 'household', 'households', 'houses', 'housing', 'houten', 'howard', 'hpc', 'hplc', 'hpv', 'hris', 'hsv', 'hta', 'htmapril', 'html', 'http', 'https', 'huang', 'huawai', 'hubbard', 'hubble', 'hubs', 'hudson', 'hughes', 'hui', 'human', 'humane', 'humanities', 'humanity', 'humanization', 'humans', 'humboldt', 'humor', 'humoral', 'humphrey', 'hundreds', 'hunters', 'hunting', 'huntington', 'hurley', 'hurleyassistant', 'hurricane', 'hurricanes', 'husbandry', 'hybrid', 'hybridization', 'hybridized', 'hybridoma', 'hydration', 'hydraulic', 'hydrocarbon', 'hydrocarbons', 'hydrodynamics', 'hydroelectric', 'hydrogen', 'hydrogeologic', 'hydrogeology', 'hydrograph', 'hydrologic', 'hydrological', 'hydrologistusda', 'hydrology', 'hydrophytic', 'hydroponic', 'hydroxy', 'hygiene', 'hygienehttp', 'hyperchem', 'hyperplasia', 'hypertrophy', 'hypo', 'hypochlorite', 'hypotheses', 'hypothesis', 'hypothesized', 'hywywuapril', 'iaas', 'iacuc', 'iaq', 'ibeverly', 'ibm', 'ibminsight', 'ibmspeaker', 'ibrd', 'ica', 'icas', 'icd', 'ice', 'ich', 'icp', 'icr', 'ics', 'ict', 'icycler', 'ide', 'idea', 'ideas', 'identical', 'identification', 'identifications', 'identified', 'identifier', 'identifiers', 'identify', 'identifying', 'identity', 'ides', 'idl', 'ids', 'idunlap', 'ied', 'ieee', 'iemba', 'iep', 'iex', 'iformbuilder', 'iga', 'igg', 'ignite', 'igor', 'iia', 'iibiology', 'iii', 'iiidana', 'iiistate', 'iir', 'iiuniversity', 'iixoma', 'ijamtp', 'ijercse', 'ijo', 'ikonos', 'iladditional', 'ileene', 'iliescu', 'iliescur', 'illegal', 'illicit', 'illinois', 'illness', 'illumina', 'illustration', 'illustrator', 'ilson', 'imac', 'image', 'imagej', 'imagereadywork', 'imagers', 'imagery', 'images', 'imagilin', 'imaginative', 'imagine', 'imaging', 'imeche', 'immediate', 'immigration', 'immobilization', 'immune', 'immunization', 'immunizations', 'immunized', 'immuno', 'immunoassay', 'immunoassays', 'immunodot', 'immunogenic', 'immunogenicity', 'immunological', 'immunology', 'immunologyyale', 'immunopathology', 'immunosorbent', 'immunotoxin', 'immunotoxins', 'impact', 'impacting', 'impacts', 'impaired', 'impediments', 'imperatives', 'impervious', 'implement', 'implementation', 'implementationo', 'implemented', 'implementing', 'implications', 'import', 'importance', 'important', 'importing', 'imports', 'impressions', 'improve', 'improved', 'improvement', 'improvements', 'improving', 'imt', 'inactivation', 'inassembling', 'incbridport', 'inccolchester', 'inception', 'inch', 'incidence', 'incidences', 'incident', 'incidents', 'incisive', 'include', 'included', 'includes', 'including', 'inclusion', 'incmorrisville', 'income', 'incoming', 'incorporate', 'incorporated', 'incorporating', 'incorporation', 'increase', 'increased', 'increases', 'increasing', 'incredible', 'incredibly', 'incremental', 'incremented', 'incsouth', 'incubation', 'incubator', 'incubators', 'incurred', 'incwhite', 'incwilliston', 'ind', 'independence', 'independent', 'independently', 'indesign', 'index', 'india', 'indian', 'indiana', 'indianapolis', 'indicated', 'indicates', 'indications', 'indicator', 'indicators', 'indigenous', 'indirect', 'individual', 'individualized', 'individuals', 'indoe', 'indonesia', 'indoor', 'indrinking', 'induce', 'induced', 'induces', 'inducible', 'inducing', 'inducted', 'induction', 'industrial', 'industries', 'industry', 'industryo', 'ineducational', 'infect', 'infection', 'infections', 'infectious', 'infer', 'inference', 'infest', 'infestans', 'infestanshttp', 'infestation', 'infested', 'infiltration', 'infinitely', 'inflammation', 'inflammatory', 'inflated', 'inflicted', 'influence', 'influenced', 'influences', 'influencing', 'influential', 'influenza', 'informal', 'informally', 'informants', 'informatics', 'information', 'informational', 'informationcore', 'informationdata', 'informationfull', 'informationi', 'informationit', 'informationkey', 'informed', 'informing', 'infosec', 'infrared', 'infrastructure', 'ingredients', 'inhabited', 'inheritance', 'inhibition', 'inhibits', 'initial', 'initiate', 'initiated', 'initiating', 'initiation', 'initiative', 'initiatives', 'initiator', 'injected', 'injection', 'injections', 'injector', 'injured', 'injury', 'inks', 'inmate', 'inmates', 'inn', 'inner', 'innew', 'innovation', 'innovations', 'innovative', 'innovators', 'innwilliston', 'inoculation', 'inorganic', 'inpatient', 'inpatients', 'input', 'inputs', 'inquiries', 'inquiry', 'inresearch', 'insect', 'insecticide', 'insects', 'inside', 'insider', 'insight', 'insightproject', 'insights', 'inspected', 'inspection', 'inspections', 'inspector', 'inspectors', 'inspiration', 'inspire', 'inspired', 'insr', 'install', 'installation', 'installations', 'installed', 'installing', 'installs', 'instant', 'instars', 'instigation', 'institiute', 'institut', 'institute', 'instituted', 'institutes', 'institutional', 'institutions', 'instituto', 'instron', 'instruct', 'instructed', 'instructing', 'instruction', 'instructional', 'instructions', 'instructor', 'instructornorth', 'instructors', 'instrument', 'instrumental', 'instrumentation', 'instruments', 'insurance', 'insurances', 'insure', 'insuring', 'int', 'intake', 'integr', 'integraciboõ', 'integral', 'integrate', 'integrated', 'integrates', 'integrating', 'integration', 'integrative', 'integrity', 'intel', 'intellectual', 'intelligence', 'intelligent', 'intelligently', 'intellitactics', 'intended', 'intensity', 'intensive', 'inter', 'interact', 'interacted', 'interacting', 'interaction', 'interactions', 'interactive', 'interactively', 'interacts', 'interagency', 'interconnection', 'interested', 'interesting', 'interesto', 'interests', 'interface', 'interfaced', 'interfaces', 'interfacing', 'interference', 'interim', 'interior', 'interleukin', 'intermediate', 'intern', 'internadidas', 'internal', 'internalization', 'international', 'internationally', 'internavalon', 'interncommunity', 'interndynamica', 'interned', 'interneigen', 'internemsl', 'internet', 'internglens', 'internhonda', 'internmc', 'internnavinet', 'internnorthern', 'internpioneer', 'internradiology', 'internransom', 'internrhode', 'interns', 'internship', 'internshipove', 'internshipsound', 'internspatial', 'internstate', 'internsubway', 'interntional', 'internu', 'internvanasse', 'internworking', 'interpersonal', 'interpret', 'interpretation', 'interpretations', 'interpreted', 'interpreter', 'interpreting', 'interruptions', 'interspersed', 'intervals', 'intervention', 'interventional', 'interventions', 'interview', 'interviewed', 'interviewers', 'interviewing', 'interviews', 'intima', 'intimal', 'intimate', 'intracervical', 'intramuscular', 'intranasal', 'intrepretation', 'intro', 'introduce', 'introduced', 'introducing', 'introduction', 'introductory', 'intuitive', 'invasive', 'invent', 'invented', 'inventions', 'inventoried', 'inventories', 'inventory', 'inventorying', 'inversion', 'inverter', 'investigaciones', 'investigate', 'investigated', 'investigating', 'investigation', 'investigational', 'investigations', 'investigative', 'investigator', 'investigators', 'investigatorsu', 'investment', 'investments', 'investor', 'investors', 'invitation', 'invited', 'invoice', 'invoices', 'involve', 'involved', 'involvedall', 'involvement', 'involving', 'iodination', 'ion', 'ios', 'iot', 'iowa', 'iown', 'ipa', 'ipad', 'ipid', 'ipl', 'ipod', 'ips', 'ipswich', 'ipx', 'ipython', 'ira', 'iraqi', 'irb', 'irbs', 'irobot', 'irobotcorp', 'iron', 'irradiation', 'irreplaceable', 'irving', 'irvington', 'isabella', 'isc', 'isco', 'island', 'iso', 'isocenter', 'isoelectric', 'isolate', 'isolated', 'isolating', 'isolation', 'isotonic', 'isotope', 'isotopic', 'israel', 'iss', 'issuance', 'issue', 'issued', 'issues', 'isthmus', 'italian', 'italy', 'itc', 'itcooõ_rdinator', 'item', 'items', 'iterations', 'iterative', 'ithaca', 'itk', 'itunes', 'ivi', 'ivis', 'ivu', 'iwaterbury', 'iweer', 'jackson', 'jacob', 'jacobus', 'jaffrey', 'jake', 'james', 'jamestown', 'jan', 'january', 'janurary', 'japan', 'japanese', 'japonica', 'jaslow', 'jasmine', 'jason', 'java', 'javaadditional', 'javascript', 'javascripts', 'jay', 'jaypeakresort', 'jbc', 'jcaho', 'jct', 'jdbc', 'jdk', 'jeff', 'jefferson', 'jeffersonville', 'jennifer', 'jericho', 'jersey', 'jerseys', 'jessica', 'jetbrains', 'jewett', 'jiaotong', 'jigme', 'jim', 'jira', 'jmp', 'jms', 'job', 'jobs', 'jobsnorwich', 'joe', 'john', 'johns', 'johnsbury', 'johnson', 'johnsonprovide', 'join', 'joined', 'joining', 'joint', 'jointly', 'jones', 'jong', 'jordan', 'jos', 'jose', 'joseph', 'journal', 'journals', 'jpa', 'jpql', 'jquery', 'jsf', 'json', 'jsp', 'jubilant', 'judicial', 'judith', 'juggling', 'jul', 'julia', 'july', 'julythis', 'jun', 'junction', 'june', 'jungle', 'junior', 'junit', 'juno', 'jurisdictional', 'jurisdictions', 'jyoung', 'kaizen', 'kalam', 'kalamazoo', 'kali', 'kalman', 'kana', 'kane', 'kanecompliance', 'kappa', 'karat', 'karhunen', 'karl', 'karnataka', 'karouna', 'katheryn', 'katrina', 'kayaking', 'kazal', 'kearns', 'keen', 'keene', 'keeping', 'keepingshipping', 'keeps', 'kegging', 'keithly', 'kelley', 'kelliher', 'kelly', 'kelvin', 'kemp', 'kenai', 'kendo', 'kennedy', 'kennedyresearch', 'kenneth', 'kensico', 'kensington', 'kent', 'kentucky', 'kenyaconducted', 'kept', 'kerley', 'kernel', 'kerry', 'kestrels', 'keurig', 'kevex', 'key', 'keybank', 'keys', 'kgaa', 'kgf', 'kgfr', 'khalilieh', 'kibana', 'kidney', 'kids', 'killing', 'kills', 'kiln', 'kilns', 'kilometers', 'kim', 'kimball', 'kinase', 'kind', 'kindle', 'kindness', 'kinetoplastida', 'kinetoplastids', 'kingdom', 'kingston', 'kinship', 'kirby', 'kissing', 'kit', 'kitchen', 'kits', 'kittell', 'kiva', 'klallam', 'klein', 'kleincastleton', 'klepner', 'klinger', 'klt', 'knack', 'knee', 'knock', 'knockout', 'know', 'knowledge', 'knowledgeable', 'known', 'knoxville', 'kocieba', 'kodak', 'kodiak', 'kokkinos', 'kol', 'korea', 'korean', 'kpi', 'kraft', 'krauss', 'kronenberg', 'kruzel', 'kslincoln', 'kuchroo', 'kujawa', 'kujawasenior', 'kull', 'kurt', 'kurth', 'kurthresearch', 'lab', 'labbms', 'labdaq', 'labdata', 'label', 'labeled', 'labeling', 'labelled', 'labels', 'labor', 'laboratories', 'laboratory', 'laboratorylinks', 'labs', 'labscolchester', 'labsolutions', 'labsuniversity', 'labview', 'labyrinth', 'lachat', 'lack', 'lacontinuing', 'lactoferrin', 'laderach', 'lafayette', 'lageophysicist', 'lagor', 'lagorburlington', 'lake', 'lakecalifornia', 'lakes', 'lambda', 'lamblia', 'lamda', 'lamoille', 'lamp', 'lamprey', 'lamps', 'lanagues', 'lance', 'land', 'landed', 'landfill', 'landowners', 'landreth', 'lands', 'landsat', 'landscape', 'landscapes', 'landscaping', 'lane', 'language', 'languages', 'lankahuasa', 'laptop', 'laqa', 'laresearch', 'large', 'larger', 'largest', 'largestnon', 'larosiliere', 'larval', 'laser', 'lasting', 'late', 'latent', 'latest', 'lathes', 'latin', 'lattice', 'laude', 'lauderdale', 'launch', 'launched', 'launching', 'launchlogistics', 'laura', 'lauten', 'lav', 'law', 'lawn', 'lawrence', 'laws', 'laxi', 'lay', 'layer', 'laying', 'layout', 'layouts', 'lazio', 'lbs', 'lcd', 'lcms', 'lcmsms', 'lda', 'lea', 'leachate', 'lead', 'leaded', 'leader', 'leaderbio', 'leadercampus', 'leaderkeybank', 'leaders', 'leadership', 'leading', 'leads', 'leaf', 'leak', 'lean', 'learn', 'learned', 'learnedthat', 'learner', 'learning', 'learningo', 'learns', 'leave', 'lebanon', 'leco', 'lecture', 'lecturer', 'lecturersbrr', 'lectures', 'led', 'lee', 'leeman', 'legacy', 'legal', 'legislation', 'legislator', 'legislature', 'legnano', 'lehmann', 'leisure', 'lenahan', 'lenck', 'lending', 'lengthy', 'lentivirus', 'leonid', 'lesley', 'lesson', 'lessons', 'let', 'letcher', 'letter', 'letters', 'leucoraja', 'leukemia', 'leveille', 'level', 'levels', 'levelsudbury', 'leverage', 'leveraged', 'leverages', 'leveraging', 'levi', 'levied', 'lewis', 'lewiston', 'lexington', 'liability', 'liaised', 'liaison', 'liasion', 'liberal', 'liberia', 'libertyville', 'librarian', 'libraries', 'library', 'libreoffice', 'license', 'licensed', 'licensejanuary', 'licenses', 'licensesacls', 'licensesaemtmay', 'licensescpr', 'licensesdriver', 'licenseslean', 'licensing', 'lichtler', 'lidar', 'lieberherr', 'life', 'lifecycle', 'lifelong', 'lift', 'ligands', 'light', 'lights', 'lightweight', 'like', 'likelihood', 'likes', 'liking', 'limate', 'limit', 'limitation', 'limited', 'lims', 'lin', 'lincoln', 'linda', 'lindsey', 'line', 'lineages', 'linear', 'linearization', 'linearizers', 'linearly', 'lines', 'linguistics', 'lining', 'link', 'linked', 'linkedin', 'linking', 'linkless', 'links', 'linux', 'lip', 'lipton', 'liquid', 'liquids', 'lis', 'lisbon', 'lisp', 'list', 'listed', 'listening', 'listing', 'lists', 'literacy', 'literary', 'literate', 'literature', 'lithography', 'lithographyibm', 'lithuania', 'litigation', 'little', 'live', 'livelihood', 'livelihoods', 'liver', 'lives', 'livestock', 'livewire', 'living', 'llc', 'load', 'loaded', 'loading', 'loads', 'loans', 'lobbied', 'lobe', 'local', 'localities', 'locality', 'localization', 'localized', 'locally', 'locate', 'located', 'locating', 'location', 'locations', 'locator', 'lock', 'locke', 'lockehead', 'lockheed', 'locknar', 'lodge', 'loess', 'loeõ', 'log', 'logan', 'logbooks', 'logger', 'loggers', 'logging', 'logic', 'logical', 'logistic', 'logistical', 'logistics', 'logo', 'logs', 'lolo', 'lompoc', 'long', 'longer', 'longevity', 'longitudinal', 'look', 'looked', 'looking', 'los', 'losch', 'loschburlington', 'loss', 'losses', 'lossless', 'lossy', 'lost', 'lot', 'lotions', 'lotus', 'loud', 'loudness', 'louis', 'louisiana', 'lout', 'loutecologist', 'low', 'lowell', 'lower', 'lowest', 'loyola', 'lps', 'lso', 'ltq', 'lts', 'lucero', 'lucideus', 'luciferase', 'lucy', 'ludewig', 'luis', 'lukes', 'luna', 'lunch', 'luncheon', 'lung', 'lyme', 'lymphocyte', 'lyndonville', 'lynn', 'lynnfield', 'lyophilizer', 'lysate', 'lysine', 'maadditional', 'maauthorized', 'mab', 'mabs', 'mac', 'macaque', 'macaques', 'mach', 'machamplain', 'machine', 'machinelearning', 'machinery', 'machines', 'machining', 'machinist', 'macintosh', 'macintoshos', 'macomb', 'macontent', 'macro', 'macros', 'macvector', 'mad', 'madame', 'maddox', 'madigan', 'madison', 'mae', 'magazine', 'magnets', 'magnification', 'magnitude', 'magnolia', 'mahajana', 'mahoney', 'mail', 'mailed', 'mailers', 'mailings', 'mailmerges', 'mails', 'main', 'maine', 'mainframe', 'mainframes', 'mainly', 'maintain', 'maintainable', 'maintained', 'maintainer', 'maintaining', 'maintains', 'maintenance', 'major', 'majoring', 'majority', 'majors', 'makers', 'makes', 'making', 'malaria', 'maldi', 'male', 'malfunction', 'malfunctioning', 'malia', 'malignant', 'malone', 'malware', 'mamelrose', 'mammalian', 'mammalogy', 'mammals', 'mammary', 'man', 'manage', 'managed', 'management', 'managementfood', 'managementgreen', 'managementhigh', 'managementhome', 'managementwork', 'manager', 'managerbarn', 'managerburgess', 'managercharged', 'managerchooseco', 'managercm', 'managercns', 'managercorning', 'managerdesmond', 'managerearth', 'managerfairvue', 'managerhoyle', 'managerial', 'manageribm', 'managerliquid', 'managernew', 'managernorthern', 'manageromega', 'managerporter', 'managerquapaw', 'managerregional', 'managers', 'managersaint', 'managerseldon', 'managerst', 'managerthe', 'managerverde', 'managerwestern', 'managerwhite', 'managerworld', 'managerxoma', 'manages', 'managing', 'manchester', 'mandarin', 'mandated', 'maneuver', 'manganese', 'manhattan', 'mania', 'manila', 'manipulate', 'manipulated', 'manipulating', 'manipulation', 'manipulations', 'manipulator', 'manner', 'manor', 'mansfield', 'manual', 'manually', 'manuals', 'manufacture', 'manufactured', 'manufacturer', 'manufacturers', 'manufacturing', 'manure', 'manuscript', 'manuscripts', 'map', 'mapk', 'maple', 'mapp', 'mapped', 'mapping', 'mappinglinks', 'maprofessional', 'maps', 'mar', 'marathon', 'marbled', 'marcellino', 'march', 'marcole', 'marcus', 'margaret', 'margin', 'margolin', 'maria', 'marie', 'mariel', 'marine', 'maritime', 'mark', 'marked', 'marker', 'markers', 'market', 'marketed', 'marketing', 'markets', 'marking', 'markov', 'marlborough', 'mars', 'marsh', 'marshall', 'martel', 'martial', 'martian', 'martin', 'martingale', 'marwan', 'mary', 'maryland', 'marylandtaught', 'mascot', 'mashelkar', 'mask', 'maslt', 'mass', 'massachusetts', 'massage', 'masshunter', 'mast', 'master', 'masterfoods', 'mastering', 'masters', 'mastitis', 'match', 'matched', 'matches', 'matching', 'material', 'materials', 'maternal', 'maternity', 'math', 'mathematica', 'mathematical', 'mathematician', 'mathematics', 'mathematicsthe', 'maths', 'matlab', 'matlabmember', 'matrix', 'matt', 'matter', 'matters', 'matthew', 'mature', 'maven', 'mawli', 'maximization', 'maximize', 'maximizing', 'maximum', 'maynard', 'mayo', 'mba', 'mbf', 'mcb', 'mccann', 'mccue', 'mccullen', 'mccullough', 'mcenroe', 'mcenroemonkton', 'mcgrew', 'mcgrewpresident', 'mckenna', 'mckennachief', 'mclean', 'mcmc', 'mcnair', 'mcs', 'mct', 'mdd', 'mdresearch', 'mead', 'meadows', 'meal', 'meals', 'mean', 'meaningful', 'means', 'meanshift', 'measurable', 'measurableo', 'measure', 'measured', 'measurement', 'measurements', 'measures', 'measuring', 'meat', 'mechanical', 'mechanicals', 'mechanics', 'mechanicssolid', 'mechanism', 'mechanisms', 'med', 'medallion', 'medals', 'media', 'mediaevent', 'medias', 'mediated', 'mediation', 'medicaid', 'medical', 'medically', 'medicare', 'medication', 'medications', 'medicine', 'medicineproject', 'medicinesouth', 'meditech', 'medium', 'medra', 'meegid', 'meehl', 'meet', 'meeting', 'meetings', 'meetingsrelated', 'meets', 'mega', 'megan', 'megawatt', 'meghan', 'mehren', 'melekos', 'melissa', 'mellon', 'melrose', 'melrosepublic', 'melts', 'melville', 'member', 'memberfluor', 'memberpeter', 'members', 'membership', 'membrane', 'memorial', 'mendez', 'mendham', 'menlo', 'menopausal', 'mental', 'mentee', 'mention', 'mentor', 'mentored', 'mentoring', 'mentors', 'menu', 'meo', 'merchandise', 'merchants', 'merck', 'mercury', 'merged', 'merging', 'merit', 'meru', 'mesenchymal', 'meskillsnetwork', 'message', 'messages', 'messaging', 'met', 'meta', 'metabolic', 'metabolism', 'metabolite', 'metabolites', 'metagenomic', 'metagenomics', 'metagenomicsrna', 'metal', 'metals', 'metamorph', 'metastasis', 'metastatic', 'meteor', 'meteorological', 'meter', 'metering', 'meters', 'methadone', 'methcathinone', 'method', 'methodical', 'methodo', 'methodologies', 'methodology', 'methodresearch', 'methods', 'methodsignal', 'methodsresearch', 'methodsthe', 'methodswe', 'meticulous', 'metric', 'metrics', 'metricscomputer', 'metrologist', 'metrologisteast', 'metrology', 'metropolitana', 'mexican', 'mexico', 'meyers', 'mfg', 'mgh', 'mianus', 'mice', 'michael', 'michelle', 'michigan', 'michrom', 'micr', 'micro', 'microarray', 'microarrays', 'microbes', 'microbial', 'microbiol', 'microbiological', 'microbiologist', 'microbiology', 'microbiologyof', 'microbiome', 'microbiomes', 'microcal', 'microcavitation', 'microchip', 'microcode', 'microfilm', 'microgridîâ', 'microimaging', 'microorganisms', 'microparticle', 'microphone', 'microphones', 'micropipetting', 'microplate', 'microscope', 'microscopes', 'microscopic', 'microscopist', 'microscopu', 'microscopy', 'microscopyled', 'microsoft', 'microsoftîâ', 'microstructure', 'microsystems', 'microtome', 'micrsocopy', 'mid', 'middle', 'middlebury', 'middleburynew', 'middlesex', 'midi', 'midreshet', 'midwinter', 'migraine', 'migrate', 'migration', 'migrations', 'migratory', 'miguel', 'mike', 'mile', 'mileage', 'miles', 'milestoneso', 'milford', 'military', 'miliusself', 'milk', 'milking', 'millbrook', 'millburn', 'millennium', 'miller', 'millfibers', 'million', 'millions', 'mills', 'milton', 'milwaukee', 'mimic', 'mimicking', 'mind', 'mindful', 'minds', 'mindset', 'mineral', 'minerals', 'mines', 'minimal', 'minimally', 'minimax', 'minimize', 'mining', 'ministry', 'minitab', 'minitabtm', 'minor', 'minority', 'minorscolumbia', 'minutes', 'mirrors', 'mis', 'misses', 'missing', 'mission', 'missions', 'missoula', 'mist', 'misuse', 'mit', 'mitch', 'mitchell', 'mitigate', 'mitigating', 'mitigation', 'mitochondrial', 'mitre', 'mixed', 'mixing', 'mixture', 'mixtures', 'miyaura', 'mls', 'mngmnt', 'mobile', 'mobileceo', 'mobility', 'mobilization', 'mobilizations', 'mobilized', 'moc', 'mockito', 'mod', 'modalities', 'mode', 'model', 'modeled', 'modeler', 'modelestimation', 'modeling', 'models', 'modelsguest', 'modelsresearch', 'moderate', 'moderately', 'moderatoro', 'modern', 'modernization', 'modicon', 'modifiable', 'modification', 'modifications', 'modified', 'modifiers', 'modify', 'modulating', 'module', 'modules', 'moi', 'moiety', 'moisture', 'moisturizers', 'mokito', 'mol', 'molbiolcell', 'mold', 'molding', 'molecular', 'molecule', 'molecules', 'mombassa', 'moment', 'monahan', 'monahanintern', 'monetary', 'money', 'mongodb', 'monies', 'monitor', 'monitored', 'monitoring', 'monitors', 'monkey', 'mono', 'monochromatic', 'monoclonal', 'monographs', 'monophyly', 'monroe', 'montage', 'montana', 'monte', 'month', 'monthly', 'months', 'monthsrstudy', 'montpelier', 'montreõ', 'mood', 'mooney', 'mopac', 'morale', 'moreeasily', 'morgan', 'morgantown', 'morphology', 'morris', 'morrisville', 'morse', 'mortgage', 'mos', 'mosaic', 'mosaics', 'moscow', 'mosquitoes', 'mother', 'motif', 'motifs', 'motile', 'motility', 'motion', 'motions', 'motivated', 'motivating', 'motivation', 'mount', 'mountain', 'mountainside', 'mounted', 'mounting', 'mouse', 'moved', 'movement', 'movements', 'moving', 'mowed', 'mowing', 'mpa', 'mpn', 'mri', 'mrna', 'mrp', 'mrs', 'msc', 'msds', 'msms', 'msn', 'msvisual', 'msx', 'mtn', 'mtt', 'mucus', 'mudpuppy', 'muffle', 'mukherjee', 'mulheron', 'mulit', 'mulitparameter', 'multi', 'multicolor', 'multilateral', 'multimedia', 'multimodal', 'multinational', 'multiparameter', 'multiphoton', 'multiple', 'multiplex', 'multisensor', 'multispectral', 'multitasking', 'multithreaded', 'multithreading', 'multiuser', 'multivariate', 'mumbai', 'municipal', 'municipalities', 'municipality', 'munson', 'murine', 'murray', 'muscle', 'muscles', 'muscular', 'museum', 'mushra', 'music', 'musical', 'musicians', 'mussels', 'muta', 'mutagenesis', 'mutagenicity', 'mutants', 'mutating', 'mutations', 'mutual', 'mvc', 'mvcsoftware', 'mvp', 'mvs', 'mycology', 'myeclipse', 'myeloid', 'myer', 'myocardial', 'myotis', 'myprofessional', 'myresearch', 'mysore', 'mysql', 'mysqlusing', 'mytenure', 'naaee', 'nacional', 'nadu', 'nadumasters', 'nagios', 'naiõ_ve', 'nalbant', 'naming', 'nancy', 'nano', 'nanoflow', 'nanomaterial', 'nanomaterials', 'nanoparticle', 'nanoparticles', 'nanotubes', 'nanyou', 'narrow', 'nasa', 'nashville', 'natick', 'nation', 'national', 'nationally', 'nations', 'nationsnew', 'nationwide', 'native', 'nato', 'natrual', 'natural', 'naturalists', 'naturally', 'nature', 'naturescience', 'nautical', 'nav', 'navair', 'naval', 'navigable', 'navigate', 'navigated', 'navigation', 'navigator', 'navinet', 'navsea', 'navy', 'nawras', 'nawrasabureehan', 'ncbi', 'ncchapel', 'nccls', 'ncircle', 'ndglurs', 'ndvi', 'near', 'nearby', 'nearly', 'nebraska', 'necap', 'necessary', 'necessity', 'necla', 'need', 'needed', 'neededoffice', 'needing', 'needs', 'negative', 'negev', 'neglect', 'negotiate', 'negotiated', 'negotiating', 'negotiation', 'negotiations', 'negotiator', 'neighborhood', 'neighbors', 'neil', 'nem', 'nemac', 'nemethy', 'nemx', 'neo', 'neoadjuvant', 'nephew', 'nervous', 'nes', 'nest', 'nesting', 'nestlings', 'nests', 'net', 'netball', 'netbeans', 'netdraw', 'netherlands', 'nets', 'netted', 'netting', 'network', 'networked', 'networking', 'networks', 'networkso', 'neural', 'neuro', 'neuroanatomy', 'neurobiological', 'neurobiology', 'neuroendocrine', 'neuroimaging', 'neurological', 'neurologists', 'neurology', 'neurolucida', 'neuron', 'neuronal', 'neurons', 'neurophysiology', 'neuropsychology', 'neuroscience', 'neurospora', 'neurosurgery', 'neutral', 'neutrophil', 'neutrophils', 'nevadafamily', 'nevercookie', 'new', 'newcastle', 'newly', 'newman', 'newport', 'news', 'newsletter', 'newsletters', 'newwork', 'nextlevel', 'neyman', 'nfs', 'ngep', 'ngo', 'nhcommand', 'nhelectrodes', 'niaaa', 'nicaragua', 'nicholas', 'nicksindorf', 'nicolaus', 'nidup', 'night', 'nightly', 'nights', 'nighttime', 'nih', 'nikon', 'nino', 'ninomedical', 'niple', 'nis', 'nisha', 'nist', 'nitrate', 'nitrates', 'nitrazine', 'nitric', 'nitrite', 'nitrogen', 'njclinical', 'njresearch', 'nlm', 'nmds', 'nmr', 'nocturnal', 'node', 'nodes', 'nodeso', 'noida', 'noise', 'nolij', 'nominal', 'nominated', 'non', 'nonadjuvanted', 'nonlinear', 'nonparametric', 'nonspecific', 'noran', 'nordensonpolice', 'normal', 'norsworthy', 'north', 'northamerican', 'northeast', 'northeastcns', 'northeastern', 'northern', 'northernother', 'northfield', 'northwestern', 'norwalk', 'norwegian', 'norwich', 'norwood', 'nose', 'notarity', 'notary', 'note', 'notebook', 'notebooks', 'noted', 'notepad', 'notes', 'noteworthy', 'notice', 'notifications', 'nourishment', 'nov', 'nova', 'novasoft', 'novel', 'novell', 'novels', 'november', 'novo', 'noõ', 'npd', 'nps', 'nrec', 'nrm', 'nsa', 'nsc', 'nsf', 'nsts', 'nti', 'nuclear', 'nucleic', 'nucleus', 'nude', 'nugen', 'nuisance', 'nulhegan', 'null', 'number', 'numbering', 'numbers', 'numerical', 'numerous', 'numpy', 'nunyunsthe', 'nurse', 'nurseberwich', 'nursefairfax', 'nursemansfield', 'nursemaple', 'nursemhm', 'nursemobile', 'nurseries', 'nurserobert', 'nursery', 'nurses', 'nursing', 'nursingpace', 'nursingvermont', 'nurture', 'nurtured', 'nutrient', 'nutrients', 'nutrition', 'nutritional', 'nutritionals', 'nwi', 'nyadditional', 'nyassisted', 'nyassociates', 'nyauthorized', 'nybachelor', 'nyc', 'nylinks', 'nymanagement', 'nymicrosoft', 'nymph', 'nymphal', 'nyny', 'nyr', 'nysoftware', 'nysupervising', 'nyworked', 'nõûez', 'oak', 'oakham', 'oakhamian', 'oakland', 'oasis', 'object', 'objective', 'objectivec', 'objectively', 'objectives', 'objects', 'obligations', 'observation', 'observational', 'observations', 'observe', 'observed', 'obsessed', 'obsolescence', 'obstetrics', 'obtain', 'obtained', 'obtaining', 'obvious', 'occasional', 'occasions', 'occupancy', 'occupant', 'occupational', 'occupied', 'occur', 'occurred', 'occurring', 'ocean', 'oceanographic', 'ochs', 'ocms', 'oct', 'october', 'odd', 'oecd', 'oem', 'oems', 'offender', 'offer', 'offered', 'offering', 'offerings', 'offers', 'office', 'officer', 'officercity', 'officeremployed', 'officermimosa', 'officernorwich', 'officers', 'officerstarfire', 'officerstate', 'officertown', 'offices', 'officials', 'offline', 'offset', 'offshore', 'offspring', 'ofits', 'ofsystem', 'ohbachelor', 'ohio', 'oil', 'oiling', 'ojt', 'okan', 'okelly', 'okinawa', 'oklahoma', 'olap', 'old', 'olefin', 'oligomeric', 'ols', 'olsen', 'olympiad', 'olympus', 'omaha', 'omega', 'omim', 'onappropriate', 'onboarding', 'oncogene', 'oncogenes', 'oncol', 'oncologic', 'oncology', 'oncologyfox', 'ones', 'ongoing', 'online', 'onlinelibrary', 'onsite', 'onsoftware', 'ontology', 'ooad', 'open', 'opencv', 'opened', 'opengl', 'opening', 'openings', 'openinventor', 'operate', 'operated', 'operating', 'operation', 'operational', 'operations', 'operationsibm', 'operative', 'operator', 'operatorbirds', 'operatorcity', 'operatorgeorge', 'operatoribm', 'operators', 'operatorspatial', 'operatorstate', 'opiates', 'opinion', 'opinions', 'opportunities', 'opportunity', 'opsonized', 'optical', 'optics', 'opticsdirector', 'optimal', 'optimization', 'optimizations', 'optimize', 'optimized', 'optimizing', 'optimum', 'option', 'options', 'optionso', 'oracle', 'oraclesoftware', 'oral', 'orally', 'oram', 'orange', 'orbiter', 'orcad', 'orchestrate', 'orchestrated', 'orchestration', 'order', 'ordered', 'ordering', 'orders', 'ordinance', 'ordinate', 'ordinates', 'ordnance', 'oregon', 'org', 'organic', 'organics', 'organisms', 'organization', 'organizational', 'organizations', 'organize', 'organized', 'organizing', 'orientation', 'orientations', 'oriented', 'orienteering', 'origin', 'original', 'originally', 'orlando', 'orleans', 'ornithological', 'orono', 'orthopaedics', 'orthopedic', 'orthophoto', 'oryza', 'osha', 'oskira', 'osol', 'osprey', 'oss', 'ossining', 'osteoarthritis', 'osteoblastic', 'ostfeld', 'oswald', 'oswego', 'osx', 'otherfruit', 'otherpersonnel', 'otherssales', 'otherworld', 'otoacoustic', 'otsu', 'ounce', 'ouro', 'outbreak', 'outbuilding', 'outcome', 'outcomes', 'outdoor', 'outgoing', 'outing', 'outings', 'outlets', 'outline', 'outlined', 'outlining', 'outlook', 'outpatient', 'outpatients', 'outpost', 'output', 'outreach', 'outreaches', 'outside', 'outstanding', 'outstandingly', 'oval', 'ovarian', 'ovariectomized', 'ovaries', 'ovary', 'ovation', 'oven', 'ovens', 'overall', 'overexpression', 'overhauled', 'overlooked', 'overnight', 'oversaw', 'overseas', 'oversee', 'overseeing', 'oversees', 'oversight', 'oversized', 'overview', 'overviews', 'oviductal', 'oviposition', 'ovipositional', 'owls', 'owned', 'owner', 'owners', 'ownership', 'oxidant', 'oxidase', 'oxidation', 'oxide', 'oxidize', 'oxygen', 'oyster', 'oysters', 'pablo', 'pace', 'paced', 'pacific', 'package', 'packaged', 'packages', 'packaging', 'packbot', 'packer', 'packet', 'packets', 'paclitaxel', 'pacu', 'pad', 'pads', 'page', 'pages', 'pah', 'paige', 'paint', 'painterchester', 'painting', 'pair', 'pairroma', 'pak', 'palatinateteam', 'palestinian', 'palisades', 'palliative', 'palm', 'palmetto', 'palmprint', 'palo', 'paltz', 'pan', 'panama', 'panamanian', 'panchromatic', 'panel', 'panels', 'pante', 'paola', 'paper', 'paperless', 'papers', 'paperwork', 'paraffin', 'paraffins', 'parallel', 'parameter', 'parameters', 'parametric', 'paramus', 'parasite', 'parasites', 'parasitic', 'parasitology', 'parasympathetic', 'parent', 'parental', 'parentheral', 'parents', 'park', 'parks', 'parkyn', 'parmigianiduke', 'parole', 'parolees', 'parsons', 'partial', 'participant', 'participants', 'participate', 'participated', 'participates', 'participating', 'participation', 'participatory', 'particle', 'particular', 'particularly', 'particulate', 'parties', 'partlow', 'partner', 'partnered', 'partnering', 'partners', 'partnership', 'partnerships', 'parts', 'party', 'parvum', 'pascal', 'pass', 'passages', 'passed', 'passenger', 'passerine', 'passes', 'passing', 'passion', 'passionate', 'passions', 'passive', 'past', 'pasta', 'pasteur', 'pastoris', 'pasture', 'patch', 'patches', 'patcheso', 'patel', 'patent', 'patented', 'patents', 'patentsgene', 'path', 'pathfinder', 'pathogen', 'pathogenic', 'pathogens', 'pathologist', 'pathologists', 'pathology', 'paths', 'pathsfair', 'pathway', 'pathways', 'patience', 'patient', 'patients', 'patlz', 'patrick', 'patrol', 'patrons', 'pattern', 'patterns', 'paul', 'pavement', 'pavements', 'pavilions', 'paving', 'pawstrucking', 'payable', 'paychex', 'payer', 'payload', 'payment', 'payments', 'payouts', 'payroll', 'pca', 'pcb', 'pcc', 'pcoffee', 'pcr', 'pcrdna', 'pcs', 'pcstest', 'pctrunning', 'pdb', 'pdf', 'pdffebruary', 'pdfmay', 'peace', 'peak', 'peaks', 'peal', 'pearl', 'pearson', 'pease', 'pedestrian', 'pediatric', 'pediatrics', 'peer', 'peers', 'pefectjob', 'pella', 'pen', 'pending', 'pendleton', 'penetrability', 'penetrometer', 'pennsylvania', 'people', 'peoplewilling', 'peptide', 'peptides', 'percentile', 'perception', 'perceptron', 'percussion', 'peregrine', 'perfect', 'perforce', 'perform', 'performance', 'performancegis', 'performed', 'performers', 'performing', 'performs', 'perfumes', 'peri', 'peridomestic', 'period', 'periodic', 'periodically', 'periodicals', 'periods', 'peripheral', 'peripherals', 'peritoneal', 'perkin', 'perkinsville', 'perl', 'permanent', 'permission', 'permissions', 'permit', 'permitability', 'permits', 'permitted', 'permittee', 'permittees', 'permitting', 'peroxisome', 'perry', 'persist', 'persistent', 'person', 'personable', 'personablework', 'personal', 'personalities', 'personality', 'personalized', 'personally', 'personnel', 'personnelmade', 'persons', 'personsystems', 'perspective', 'persuasive', 'pertaining', 'pertinent', 'pest', 'pesticides', 'pet', 'petabyte', 'pete', 'peter', 'petrapro', 'petrella', 'petrographic', 'petroleum', 'petrology', 'pets', 'petzoldt', 'peõ', 'pfc', 'pfizer', 'phadia', 'pharma', 'pharmaceutical', 'pharmaceuticals', 'pharmacological', 'pharmacology', 'pharmacologythe', 'phase', 'phased', 'phases', 'phd', 'phdpostdoctoral', 'phds', 'phenotype', 'phenotyping', 'pheromone', 'phi', 'philadelphia', 'philip', 'philippines', 'phillips', 'philosophy', 'philosophysalem', 'phimay', 'phlebotomist', 'phlebotomists', 'phlebotomy', 'phone', 'phonemic', 'phones', 'phonics', 'phosphorous', 'phosphorus', 'photo', 'photochromic', 'photocopied', 'photodynamic', 'photoelectron', 'photographic', 'photographing', 'photographs', 'photography', 'photomask', 'photometer', 'photonic', 'photonics', 'photos', 'photoshop', 'photoshopsenior', 'photoshopîâ', 'photovoltaic', 'photovoltaics', 'php', 'phpsoftware', 'phylogenetic', 'phylum', 'physical', 'physically', 'physicals', 'physician', 'physicians', 'physics', 'physicsbrown', 'physicsclemson', 'physicsguilford', 'physicsqueen', 'physicsstudent', 'physicssweet', 'physicstufts', 'physiol', 'physiologic', 'physiological', 'physiology', 'picea', 'picher', 'pichia', 'pichiapastoris', 'pick', 'picking', 'pickup', 'picture', 'piece', 'pieces', 'pierce', 'pierre', 'piezometer', 'pig', 'pigs', 'pii', 'pilot', 'piloting', 'pineapples', 'pinpoint', 'pinshow', 'pintle', 'pioneer', 'pioneered', 'pipe', 'pipeline', 'pipelines', 'pipes', 'pipets', 'pipetting', 'pipettors', 'pis', 'pitch', 'pitcher', 'pitfalls', 'pittcon', 'pittsburgh', 'pivot', 'pizza', 'pizzeria', 'place', 'placed', 'placement', 'placing', 'plainfield', 'plains', 'plan', 'planetary', 'planned', 'planner', 'planners', 'planning', 'plans', 'plant', 'plantation', 'planted', 'planting', 'plantings', 'plants', 'plantsskills', 'plaque', 'plasmid', 'plastic', 'plastics', 'plate', 'plates', 'platform', 'platforms', 'plating', 'platte', 'plattsburgh', 'played', 'player', 'playerproven', 'players', 'playing', 'plays', 'plc', 'plcs', 'plein', 'plexus', 'plm', 'ploof', 'plooflaboratory', 'plos', 'plot', 'plots', 'plurality', 'plus', 'pmd', 'pnas', 'pneumatic', 'pneumoconiosis', 'poetry', 'poets', 'point', 'points', 'poise', 'poland', 'polarity', 'polarization', 'pole', 'polemonium', 'police', 'policies', 'policiesmaster', 'policy', 'policymakers', 'poliovirus', 'polished', 'polishing', 'political', 'pollinator', 'pollutant', 'pollutants', 'polluters', 'pollution', 'poly', 'polyacrylamide', 'polycyclic', 'polyenes', 'polyfelt', 'polyhedral', 'polymer', 'polymerase', 'polymers', 'polymorph', 'polynomial', 'polypropylene', 'polytechnic', 'pon', 'pond', 'ponds', 'ponnampet', 'pool', 'pools', 'poor', 'pop', 'popular', 'population', 'populations', 'porifera', 'porous', 'porphyrin', 'port', 'portable', 'portal', 'portals', 'porter', 'portfolio', 'portfolios', 'porting', 'portion', 'portions', 'portland', 'portol', 'portsmouth', 'portuguese', 'posed', 'position', 'positional', 'positiondear', 'positioning', 'positionmollen', 'positions', 'positive', 'positively', 'positives', 'poss', 'possessing', 'possibility', 'possible', 'post', 'postal', 'postdocbrandeis', 'postdoctoral', 'poster', 'posterior', 'posters', 'postflight', 'postgis', 'postgres', 'postgresql', 'posting', 'postings', 'postitioneast', 'postpartum', 'postproduction', 'postresql', 'posts', 'potato', 'potency', 'potential', 'potentially', 'potsdam', 'pottersimon', 'pottery', 'poughkeepsie', 'poultney', 'pounds', 'pouring', 'poverman', 'poverty', 'powder', 'power', 'powerade', 'powered', 'powerfulpcs', 'powerpoint', 'powerpointîâ', 'powersaw', 'powerschool', 'ppe', 'ppt', 'practicable', 'practical', 'practicaluses', 'practice', 'practiced', 'practices', 'practicing', 'practitioner', 'practitioners', 'pradesh', 'pragmatic', 'prairie', 'pranab', 'prasanna', 'pratt', 'pre', 'precedent', 'preceptor', 'preceptors', 'precipitation', 'precise', 'precisely', 'precision', 'predation', 'predator', 'predict', 'predicted', 'predicting', 'prediction', 'predictions', 'predictionseast', 'predictive', 'predictor', 'preeminent', 'preferences', 'preferential', 'preformed', 'pregnancy', 'preliminary', 'premier', 'prep', 'preparation', 'preparations', 'preparatory', 'prepare', 'prepared', 'preparer', 'preparing', 'prepped', 'prepregnancy', 'preprocess', 'preps', 'presbyterian', 'prescribed', 'prescription', 'presence', 'present', 'presentable', 'presentadult', 'presentas', 'presentation', 'presentations', 'presentau', 'presentbureau', 'presentcreator', 'presentdaily', 'presentdesign', 'presentdesigned', 'presentdiverse', 'presentdraw', 'presentduties', 'presented', 'presentenabled', 'presentensured', 'presenter', 'presenteribm', 'presenthealth', 'presentin', 'presenting', 'presentjay', 'presentjob', 'presentkingdom', 'presentleads', 'presentlecturer', 'presentlindsey', 'presentmake', 'presentmanaged', 'presentme', 'presentmeyers', 'presentnew', 'presentone', 'presentoperate', 'presentpro', 'presentprovide', 'presentpublic', 'presentpython', 'presentrandolph', 'presentre', 'presentrecently', 'presentremote', 'presentresearch', 'presentsapling', 'presentschool', 'presentself', 'presentsenior', 'presentskills', 'presentsource', 'presentstate', 'presentsymbiont', 'presentteach', 'presenttem', 'presentthe', 'presentthis', 'presentusa', 'presentward', 'presentwork', 'preservation', 'preservative', 'preserve', 'preserving', 'president', 'presidential', 'presidentritec', 'presidentt', 'press', 'presses', 'pressing', 'pressure', 'pressures', 'prestigious', 'preto', 'prevalence', 'prevent', 'preventative', 'prevented', 'prevention', 'preventionnyc', 'preventive', 'previous', 'previously', 'price', 'prices', 'pricing', 'pride', 'primarily', 'primary', 'primate', 'prime', 'primer', 'primers', 'princeton', 'principal', 'principals', 'principle', 'principles', 'print', 'printed', 'printer', 'printers', 'printing', 'prints', 'prior', 'priorities', 'prioritieso', 'prioritize', 'prioritized', 'prioritizing', 'priority', 'prism', 'pristine', 'privacy', 'private', 'privately', 'privatization', 'prize', 'prizm', 'pro', 'proactive', 'proactively', 'probabilistic', 'probability', 'probable', 'probably', 'probation', 'probationary', 'probe', 'prober', 'probes', 'probing', 'probiotics', 'problem', 'problems', 'proc', 'procedural', 'procedure', 'procedureoval', 'procedures', 'proceduresfield', 'proceedings', 'process', 'processed', 'processes', 'processeso', 'processgraphic', 'processing', 'processingwork', 'processnetwork', 'processor', 'processors', 'proctor', 'proctored', 'procured', 'procurement', 'produce', 'produced', 'producing', 'product', 'production', 'productiongreen', 'productive', 'productivity', 'productoras', 'productores', 'products', 'productso', 'productsxoma', 'professional', 'professionalism', 'professionally', 'professionals', 'professionaluvm', 'professions', 'professor', 'professorglobal', 'professoriukb', 'professorpurdue', 'professors', 'professorschool', 'proficiencies', 'proficiency', 'proficient', 'profile', 'profiler', 'profiles', 'profileîâ', 'profiling', 'profilometer', 'profit', 'profitable', 'profitd', 'profitsfri', 'progenitor', 'prognosis', 'prognostics', 'program', 'programmability', 'programmatic', 'programmed', 'programmer', 'programmers', 'programming', 'programnorwich', 'programphi', 'programs', 'programsstudy', 'progress', 'progressive', 'project', 'projectiles', 'projection', 'projections', 'projectjacob', 'projecto', 'projectpaige', 'projects', 'projectschool', 'projectsroutine', 'projectswork', 'projectwendy', 'projectyuvaraja', 'proliferation', 'prolog', 'prolonged', 'prominent', 'promises', 'promote', 'promoted', 'promoter', 'promotes', 'promoting', 'promotion', 'promotional', 'promotions', 'pronoun', 'proof', 'proofing', 'prop', 'propagate', 'propagation', 'propane', 'propelled', 'propensity', 'proper', 'properly', 'properties', 'property', 'proportion', 'proposal', 'proposals', 'propose', 'proposed', 'proposing', 'proprietary', 'proprietor', 'propulsion', 'prose', 'prosecution', 'prospect', 'prospective', 'prospects', 'prostate', 'prosthetic', 'protect', 'protected', 'protecting', 'protection', 'protects', 'protein', 'proteins', 'proteome', 'proteomics', 'proteomicshttp', 'protocol', 'protocols', 'protocolswork', 'proton', 'prototype', 'prototyped', 'prototypes', 'prototyping', 'protozoan', 'prove', 'proven', 'provide', 'provided', 'providedfollow', 'providence', 'provider', 'providers', 'provides', 'providing', 'province', 'proving', 'provision', 'provost', 'proximity', 'proxy', 'pruning', 'pss', 'psychconsult', 'psychiatric', 'psychiatrists', 'psychiatry', 'psychoacoustic', 'psychoacoustics', 'psychologist', 'psychologists', 'psychology', 'psychologymount', 'psychologynew', 'psychologystate', 'psychophysics', 'psychotherapist', 'psychotherapy', 'pta', 'pto', 'ptsd', 'public', 'publication', 'publicationo', 'publications', 'publicly', 'publish', 'published', 'publishedwork', 'publisher', 'publishing', 'pubmed', 'puker', 'pull', 'puller', 'pulling', 'pulmicort', 'pulmonary', 'pump', 'pumping', 'pumps', 'punitive', 'puppetry', 'purchase', 'purchased', 'purchases', 'purchasing', 'purdive', 'pure', 'purification', 'purificationsds', 'purity', 'purpose', 'purposes', 'pursuant', 'pursue', 'pursued', 'pursuing', 'putting', 'pvc', 'pvt', 'pylori', 'pymol', 'pyrene', 'pyridine', 'pyspark', 'python', 'pythonpatent', 'qca', 'qgis', 'qhei', 'qiime', 'qmf', 'qpcr', 'qpcrteaching', 'qtap', 'quail', 'qualification', 'qualifications', 'qualified', 'qualify', 'qualitative', 'qualities', 'qualitiesself', 'quality', 'quantiferon', 'quantifiable', 'quantification', 'quantified', 'quantify', 'quantifying', 'quantitative', 'quantity', 'quapaw', 'quark', 'quarter', 'quarterly', 'quartet', 'quasi', 'quaternary', 'quechee', 'queries', 'query', 'questioned', 'questionnaire', 'questions', 'queues', 'quiagenîâ', 'quick', 'quickbooks', 'quickly', 'quickset', 'quickterrain', 'quiz', 'quizzes', 'quizzing', 'quote', 'quotes', 'quoting', 'rabbitmq', 'rabies', 'racc', 'race', 'rack', 'rad', 'radar', 'radiant', 'radicallymobile', 'radio', 'radiographs', 'radiological', 'radiology', 'radiotelemetry', 'radius', 'radon', 'rafael', 'rail', 'rails', 'rain', 'rainbow', 'rainforest', 'raise', 'raised', 'raising', 'ralph', 'raman', 'ramos', 'ran', 'ranch', 'ranches', 'randolph', 'random', 'randomly', 'range', 'ranges', 'ranging', 'rank', 'ranked', 'ranking', 'ranklinks', 'rantes', 'rapid', 'rapidly', 'rappels', 'rapport', 'raptor', 'rare', 'raritan', 'rat', 'rate', 'rater', 'rates', 'ratewounded', 'ratio', 'ratiometric', 'rational', 'raton', 'rats', 'rattlesnake', 'rattlesnakes', 'rattner', 'ratts', 'raw', 'rawls', 'ray', 'raymond', 'raymondstaff', 'rays', 'rbt', 'rda', 'rdbms', 'rdi', 'rdsa', 'rdsas', 'reach', 'reachability', 'reaching', 'react', 'reacting', 'reaction', 'reactionomes', 'reactions', 'reactive', 'reactjs', 'reactor', 'read', 'reader', 'readers', 'readiness', 'reading', 'readingsalem', 'reads', 'ready', 'reagents', 'real', 'realism', 'reality', 'realized', 'realm', 'realtime', 'reappraisal', 'reasoner', 'reasoning', 'reasoningfuzzy', 'reasons', 'reasonspaid', 'rebecca', 'rec', 'receipt', 'receipts', 'receivable', 'receive', 'received', 'receivers', 'receiveusgs', 'receiving', 'recent', 'recently', 'receptacles', 'reception', 'receptionist', 'receptor', 'receptors', 'recipient', 'reck', 'recognition', 'recognitiono', 'recognize', 'recognized', 'recognizing', 'recombinant', 'recommend', 'recommendation', 'recommendations', 'recommended', 'recommending', 'reconcentrate', 'reconciliation', 'reconnaissance', 'reconnections', 'reconstruction', 'record', 'recordbreaking', 'recorded', 'recorders', 'recording', 'recordkeeping', 'records', 'recovery', 'recoveryo', 'recreation', 'recruit', 'recruited', 'recruiter', 'recruiting', 'recruitment', 'recruitments', 'rectal', 'recurrence', 'recurrent', 'recycle', 'recycling', 'red', 'redesign', 'redesigning', 'redevelopment', 'redstone', 'reduce', 'reduced', 'reducedresponse', 'reduces', 'reducing', 'reduction', 'reductive', 'redundant', 'reduviidae', 'reed', 'reenacting', 'reengineering', 'refer', 'reference', 'references', 'referral', 'referred', 'referring', 'refilled', 'refine', 'refined', 'refining', 'refinishing', 'reflection', 'reflective', 'reflowo', 'reform', 'reforms', 'refractometer', 'refractory', 'refresher', 'refrigerator', 'refuge', 'refunds', 'regard', 'regarded', 'regards', 'regeneration', 'regenesisîâ', 'reggie', 'regimes', 'region', 'regional', 'regionally', 'regions', 'regioõ', 'register', 'registered', 'registration', 'registrationall', 'registrationo', 'regression', 'regressions', 'regul', 'regular', 'regularly', 'regulate', 'regulated', 'regulating', 'regulation', 'regulations', 'regulators', 'regulatory', 'rehabilitate', 'rehabilitation', 'rehau', 'reimbursement', 'reimbursements', 'reinfestation', 'reinforced', 'reinforcement', 'reining', 'reintroduction', 'reject', 'rejected', 'relapse', 'related', 'relatedhealth', 'relating', 'relation', 'relations', 'relationship', 'relationships', 'relationss', 'relative', 'relatively', 'relaunch', 'relay', 'relaying', 'release', 'released', 'relevancy', 'relevant', 'reliability', 'reliable', 'reliably', 'relias', 'relocate', 'relocated', 'relocation', 'reluctant', 'remain', 'remained', 'remaining', 'remarkably', 'remarks', 'rembered', 'remedial', 'remediate', 'remediation', 'remedy', 'remodeling', 'remote', 'remotely', 'removal', 'remove', 'render', 'rendered', 'rendering', 'renewable', 'renewables', 'renewals', 'renier', 'reno', 'renovated', 'renovation', 'renovations', 'renowned', 'renre', 'rental', 'rentals', 'renters', 'reorganized', 'reorganizing', 'repair', 'repaired', 'repairs', 'repeatability', 'repeated', 'repeating', 'repetitive', 'replace', 'replaced', 'replacement', 'replacing', 'replication', 'report', 'reported', 'reporter', 'reporters', 'reporting', 'reportlicensed', 'reports', 'reportsadjunct', 'reportsgraduate', 'repositioning', 'repositories', 'repository', 'represent', 'representation', 'representative', 'representatives', 'represented', 'representing', 'reproducing', 'reproduction', 'reproductive', 'republic', 'republicmay', 'reputable', 'reputation', 'request', 'requested', 'requests', 'require', 'required', 'requirement', 'requirements', 'requires', 'requiring', 'requisite', 'requisitions', 'res', 'resampling', 'rescue', 'rescues', 'reseaerch', 'research', 'researchbiology', 'researchdr', 'researched', 'researcher', 'researcherben', 'researcherpower', 'researchers', 'researcherswork', 'researching', 'researchncircle', 'researchsites', 'researchîâ', 'reseatch', 'resectable', 'reservation', 'reservations', 'reserve', 'reservoir', 'residant', 'residence', 'residences', 'residencies', 'resident', 'residential', 'residents', 'residual', 'residuals', 'resilience', 'resist', 'resistance', 'resistant', 'resistors', 'resmark', 'resolution', 'resolutiono', 'resolve', 'resolved', 'resolving', 'resonance', 'resort', 'resource', 'resourceful', 'resourcepuse', 'resources', 'resourcing', 'respect', 'respected', 'respecting', 'respectively', 'respiratory', 'respite', 'respond', 'responded', 'responding', 'response', 'responses', 'responsibility', 'responsible', 'responsive', 'rest', 'restaurant', 'restaurants', 'restd', 'resting', 'reston', 'restoration', 'restorations', 'restrained', 'restraining', 'restraint', 'restriction', 'result', 'resulted', 'resulting', 'results', 'resultsin', 'resultsteaching', 'resultswe', 'resume', 'resumenature', 'resumes', 'resumetheses', 'resuscitation', 'retail', 'retain', 'retained', 'retardant', 'retention', 'retinal', 'retinoic', 'retinoid', 'retort', 'retraineeibm', 'retrieval', 'retrieve', 'retrieving', 'retrofits', 'retrosynthesis', 'retroviral', 'retrovirus', 'return', 'returned', 'returning', 'returns', 'reunification', 'reusable', 'reuters', 'reutter', 'revalidation', 'reveal', 'revealed', 'reveals', 'revenue', 'revenues', 'reversals', 'reverse', 'reversion', 'review', 'reviewed', 'reviewer', 'reviewers', 'reviewing', 'reviewqc', 'reviews', 'revise', 'revised', 'revising', 'revision', 'revisions', 'revolution', 'revolutionary', 'rewarding', 'rework', 'rey', 'rfawardsara', 'rfps', 'rheumatology', 'rhinoceros', 'rho', 'rhode', 'ribachelor', 'riboprobes', 'ribosomal', 'rica', 'ricaconducted', 'rich', 'richard', 'richmond', 'rick', 'ride', 'riders', 'ridge', 'riding', 'rie', 'rieder', 'rief', 'rig', 'rigging', 'riggs', 'right', 'rigid', 'rigorous', 'rigs', 'rijndael', 'rios', 'rip', 'risk', 'risks', 'rita', 'ritter', 'ritterscientist', 'rituximab', 'river', 'riverfire', 'rivers', 'rmi', 'rna', 'rnaseq', 'rndartmouth', 'road', 'roadblocks', 'roadmap', 'roads', 'roadway', 'roan', 'robert', 'roberts', 'robertson', 'robins', 'robot', 'robotic', 'robotics', 'robots', 'robust', 'robustness', 'roche', 'rochelle', 'rochester', 'rock', 'rockclimbing', 'rocket', 'rockies', 'rocks', 'rockville', 'rockwell', 'rocky', 'rod', 'rodgers', 'rodriguez', 'roemhildt', 'rogina', 'rohs', 'roi', 'roject', 'role', 'roles', 'roll', 'roller', 'rolling', 'rollout', 'rolls', 'rolodex', 'roma', 'ron', 'ronsheim', 'roof', 'roofs', 'room', 'rooms', 'roosevelt', 'roosting', 'roosts', 'root', 'roots', 'ror', 'rose', 'rotating', 'rotatingblade', 'rotation', 'rotationberlin', 'rotations', 'rotationslittle', 'rotationsouth', 'rotavirus', 'rothermel', 'rotorcraft', 'rouge', 'rough', 'roughly', 'round', 'rounds', 'rounup', 'rouses', 'route', 'router', 'routers', 'routes', 'routine', 'routing', 'routman', 'row', 'roy', 'royal', 'royalton', 'roys', 'rpi', 'rplc', 'rrna', 'rsm', 'rsms', 'rsv', 'rtcp', 'rte', 'rti', 'rtp', 'rtsp', 'rtx', 'rubber', 'rubenstein', 'rubin', 'ruby', 'rufu', 'rugby', 'rule', 'rules', 'rumped', 'run', 'rundergraduate', 'runner', 'runnerworking', 'running', 'runs', 'runways', 'rural', 'russia', 'russian', 'rutgers', 'ruth', 'rutland', 'ryan', 'saas', 'sabre', 'safe', 'safeguards', 'safely', 'safety', 'safetymountain', 'saharan', 'saic', 'sailing', 'saint', 'sal', 'salamander', 'sale', 'sales', 'salesforce', 'salesmaven', 'saline', 'salinity', 'salmon', 'salmonella', 'salt', 'salute', 'samantha', 'samets', 'sami', 'samlagor', 'sample', 'sampler', 'samples', 'samplesself', 'sampling', 'samsung', 'samuel', 'san', 'sand', 'sandhill', 'sandwich', 'sanitary', 'sanitation', 'sanitize', 'santiago', 'santini', 'sap', 'sapling', 'sapphire', 'sara', 'sarah', 'sas', 'sass', 'satellite', 'satisfaction', 'satisfy', 'saudi', 'savage', 'savant', 'save', 'saved', 'saving', 'savings', 'savingvisual', 'sbir', 'sbrr', 'sbu', 'scala', 'scalable', 'scale', 'scales', 'scaling', 'scan', 'scanners', 'scanning', 'scap', 'scattering', 'scclemson', 'sccps', 'scenario', 'scenarios', 'scene', 'scgreensboro', 'schad', 'schadsenior', 'schedule', 'scheduled', 'scheduler', 'schedules', 'scheduling', 'schema', 'schematic', 'scheme', 'schiff', 'schizophrenia', 'schmidt', 'schmidtowner', 'schneller', 'scholar', 'scholarcommons', 'scholars', 'scholarship', 'scholastic', 'scholl', 'school', 'schoolreading', 'schools', 'schultz', 'schuster', 'schwartz', 'science', 'sciencealaska', 'sciencearcadia', 'sciencearkansas', 'sciencecollege', 'sciencedirect', 'sciencedutchess', 'sciencegraduate', 'sciencejohnson', 'sciencemaine', 'scienceoregon', 'sciencepurdue', 'sciences', 'sciencesaint', 'sciencescornell', 'sciencesduke', 'sciencesessex', 'sciencesnova', 'sciencesother', 'sciencest', 'sciencestate', 'scienceswilling', 'scienceswyeth', 'sciencethe', 'sciencewestern', 'scientific', 'scientifically', 'scientist', 'scientistabbott', 'scientistbp', 'scientistclancy', 'scientistcrrel', 'scientistdelta', 'scientistdhmc', 'scientistecs', 'scientistibm', 'scientistkeene', 'scientistkynen', 'scientistlead', 'scientistluna', 'scientistmerck', 'scientistnew', 'scientistnova', 'scientistomega', 'scientistpfizer', 'scientists', 'scientistsanofi', 'scientistsouth', 'scientiststone', 'scientistthe', 'scientistwayne', 'scientistwistar', 'scientistwyeth', 'sciex', 'scilearn', 'scipy', 'sclerosis', 'scope', 'score', 'scored', 'scores', 'scoring', 'scott', 'scout', 'scouted', 'scouts', 'scraps', 'screen', 'screened', 'screening', 'screenings', 'screens', 'script', 'scripted', 'scripting', 'scripts', 'scriptsandroid', 'scrum', 'scuba', 'sculpture', 'scx', 'sdf', 'sdk', 'sds', 'sea', 'seal', 'seamless', 'seamlessly', 'search', 'searches', 'seascape', 'season', 'seasonal', 'seasonally', 'seasoned', 'seasons', 'seat', 'seattle', 'sebastian', 'second', 'secondary', 'seconds', 'secret', 'secretary', 'secretion', 'section', 'sectional', 'sectioning', 'sections', 'sectionvermont', 'sector', 'sectors', 'secure', 'secured', 'securing', 'securities', 'security', 'securitygreen', 'securus', 'sedation', 'sediment', 'sediments', 'seed', 'seeding', 'seedlings', 'seek', 'seekers', 'seekerstaff', 'seeking', 'seen', 'segment', 'segmentation', 'segmentationo', 'segments', 'segregation', 'seguridad', 'seismic', 'seismologist', 'seismology', 'selected', 'selecting', 'selection', 'selections', 'selective', 'selenium', 'self', 'sell', 'sellers', 'selling', 'sem', 'semantics', 'semester', 'semesters', 'semi', 'semiconductor', 'semifinalist', 'seminar', 'seminars', 'seminary', 'senate', 'send', 'sending', 'senegal', 'senior', 'seniors', 'sense', 'sensed', 'sensing', 'sensitive', 'sensitivity', 'sensor', 'sensors', 'sensory', 'sent', 'separate', 'separating', 'separation', 'separations', 'separator', 'septage', 'september', 'sequence', 'sequenced', 'sequences', 'sequencher', 'sequencing', 'sequential', 'sequentially', 'sequest', 'sequestering', 'sequestration', 'sequnix', 'serial', 'series', 'serology', 'seroquel', 'seroquelî', 'serp', 'serpent', 'serum', 'serve', 'served', 'server', 'serverless', 'servers', 'serves', 'service', 'serviced', 'serviceinvoices', 'services', 'serviceservice', 'serviceshoward', 'servicesmilton', 'servicing', 'serving', 'servlet', 'servlets', 'servo', 'session', 'sessions', 'set', 'setac', 'setauket', 'seth', 'sethuraman', 'sets', 'setting', 'settings', 'setup', 'seven', 'seventh', 'severn', 'sewage', 'sewing', 'sex', 'sexual', 'sfo', 'sfr', 'sfrisbie', 'shack', 'shadi', 'shadow', 'shadowed', 'shadowing', 'shafer', 'shaftsbury', 'shampoos', 'shana', 'shannon', 'shape', 'shaped', 'share', 'shared', 'sharepoint', 'sharing', 'sharkey', 'sharon', 'sharp', 'shaves', 'shaving', 'sheds', 'sheet', 'sheets', 'shelburne', 'shelf', 'shell', 'shellfish', 'shelter', 'shelves', 'shen', 'shenzhen', 'shield', 'shift', 'shifting', 'shifts', 'shimadzu', 'ship', 'shipments', 'shipping', 'shirleyparsons', 'shock', 'shocks', 'shoe', 'shoes', 'shoot', 'shooter', 'shooting', 'shop', 'shopdevelopment', 'shopping', 'shore', 'shorebird', 'shoretel', 'short', 'shortfebruary', 'shots', 'showcase', 'showed', 'showers', 'showing', 'shows', 'shrubs', 'shut', 'shuttle', 'sib', 'sick', 'sidewalks', 'sidney', 'siel', 'siemens', 'sierra', 'sift', 'sifting', 'sights', 'sigma', 'sign', 'signal', 'signaling', 'signals', 'signature', 'signatures', 'significance', 'significant', 'significantly', 'signing', 'signs', 'sikora', 'silesquioxane', 'silk', 'sills', 'silver', 'silvio', 'similar', 'similarity', 'simple', 'simplification', 'simplified', 'simplifying', 'sims', 'simulating', 'simulation', 'simulationo', 'simulations', 'simulator', 'simulators', 'simulink', 'simultaneous', 'simultaneously', 'sincerelyjay', 'sindorf', 'sindorffounding', 'sine', 'single', 'singlehandedly', 'singleton', 'singular', 'sinofsky', 'siri', 'sirius', 'sirna', 'site', 'sites', 'sitessenior', 'siting', 'situ', 'situation', 'situations', 'size', 'sized', 'sizing', 'skate', 'skatebase', 'sketched', 'sketches', 'sketchupîâ', 'ski', 'skiing', 'skill', 'skilled', 'skillful', 'skills', 'skillsacid', 'skillsarisg', 'skillsbat', 'skillsbusiness', 'skillsc', 'skillsclinical', 'skillscontent', 'skillsdata', 'skillset', 'skillsexcel', 'skillsfluent', 'skillsforeign', 'skillslab', 'skillslabview', 'skillslanguages', 'skillslinks', 'skillsmachine', 'skillsmatlab', 'skillsmediation', 'skillsmicrosoft', 'skillsmolecular', 'skillsmotivated', 'skillsms', 'skillsmysore', 'skillsnmr', 'skillspeople', 'skillsproject', 'skillsr', 'skillssoftware', 'skillsspanish', 'skillssql', 'skillsstrong', 'skillsword', 'skillswriting', 'skin', 'slater', 'sleeping', 'slice', 'slices', 'slide', 'slides', 'slip', 'slowly', 'sludge', 'slurry', 'slusser', 'small', 'smallholder', 'smalltalk', 'smart', 'smartboard', 'smartcycler', 'smeso', 'smith', 'smooth', 'smoothly', 'sms', 'smtp', 'smu', 'snelling', 'snippets', 'snort', 'snow', 'snowboard', 'soap', 'soar', 'soc', 'soccer', 'social', 'socially', 'society', 'societyjanuary', 'socio', 'sociology', 'sociopolitical', 'sock', 'sockets', 'sodium', 'sofets', 'soft', 'softball', 'software', 'softwarestaff', 'soil', 'soils', 'soiltesting', 'soiluniversity', 'solar', 'solaris', 'sold', 'solder', 'soldering', 'sole', 'solely', 'solicit', 'solicitations', 'soliciting', 'solid', 'solids', 'solidworks', 'solopak', 'solubility', 'soluble', 'solution', 'solutions', 'solve', 'solved', 'solver', 'solvers', 'solving', 'solvingo', 'sonagashira', 'song', 'sonny', 'sonora', 'sons', 'sony', 'soon', 'sop', 'sophisticated', 'sophomore', 'soppexca', 'sops', 'sorption', 'sort', 'sorted', 'sortingjohnson', 'sos', 'sought', 'soul', 'sound', 'source', 'sourcebook', 'sourced', 'sourcefire', 'sources', 'sourcesafe', 'sourcing', 'sourcingnorwich', 'south', 'southeast', 'southeastern', 'southern', 'southwestern', 'soviet', 'sower', 'soy', 'space', 'spaces', 'spaceshuttle', 'spain', 'spandidos', 'spanish', 'spanishbrandeis', 'spanned', 'spare', 'spared', 'spark', 'sparrow', 'sparrows', 'sparse', 'spartan', 'sparverius', 'spatial', 'spc', 'spcc', 'speaker', 'speakers', 'speaking', 'spearheaded', 'spearheading', 'spears', 'spec', 'special', 'specialist', 'specialistcns', 'specialistdept', 'specialisteq', 'specialistibm', 'specialistk', 'specialistmbf', 'specialists', 'specialized', 'specializing', 'specialties', 'specialty', 'species', 'specieshttps', 'speciesnursery', 'specific', 'specifically', 'specifications', 'specificity', 'specified', 'specimen', 'specimens', 'spectra', 'spectral', 'spectrometer', 'spectrometers', 'spectrometry', 'spectroscopy', 'spectrum', 'speculative', 'speculum', 'speech', 'speed', 'spend', 'spending', 'spent', 'sperm', 'spill', 'spinedace', 'spinella', 'spinney', 'spiral', 'spirometry', 'spiropyran', 'spite', 'splice', 'spline', 'split', 'splitting', 'spm', 'spoke', 'spoken', 'sponge', 'sponges', 'sponsor', 'sponsored', 'sponsoring', 'sponsors', 'sponsorship', 'sponsorships', 'sponsorsinfosec', 'spoon', 'sport', 'sporting', 'sports', 'sportswoman', 'spot', 'spotted', 'spouse', 'sprague', 'spray', 'spraying', 'spread', 'spreadsheet', 'spreadsheets', 'spring', 'springer', 'springfield', 'sprint', 'spss', 'spssadvisory', 'sputter', 'sputtering', 'sql', 'sqlite', 'sqlitesoftware', 'sqlserver', 'square', 'squares', 'squarespace', 'squibb', 'sri', 'sribney', 'ssis', 'ssl', 'ssrs', 'stability', 'stabilization', 'stable', 'stack', 'stadheim', 'staff', 'staffing', 'staffirobot', 'staffsummer', 'stage', 'stages', 'staging', 'stained', 'staining', 'stains', 'stakeholder', 'stakeholders', 'staking', 'stall', 'stamford', 'stamina', 'stamp', 'stand', 'standard', 'standardization', 'standardized', 'standardizing', 'standards', 'standardso', 'standing', 'stands', 'stanford', 'star', 'starbucks', 'stargauge', 'start', 'started', 'startup', 'startups', 'startupvt', 'stata', 'state', 'stated', 'statemba', 'statements', 'states', 'stateso', 'statewide', 'static', 'station', 'stations', 'statistical', 'statistician', 'statisticians', 'statistics', 'stats', 'status', 'statutory', 'staunton', 'stay', 'stayhome', 'staying', 'std', 'steady', 'stealth', 'stealthwatch', 'steam', 'steep', 'steer', 'steered', 'stella', 'stem', 'stenosis', 'step', 'stephanie', 'stephen', 'steps', 'stereology', 'stereoscopic', 'sterile', 'sterility', 'sterilized', 'sterilizing', 'steritest', 'steroid', 'steroids', 'steven', 'stevenpbrady', 'stevens', 'stewardship', 'stiffness', 'stimulation', 'stitchingneuron', 'stitchingo', 'stm', 'stochastic', 'stock', 'stocked', 'stocks', 'stoichiometry', 'stomach', 'stone', 'stony', 'stop', 'storage', 'store', 'stored', 'stores', 'stories', 'storing', 'storm', 'stormwater', 'storrs', 'story', 'storyboard', 'storybooks', 'storytelling', 'strafford', 'strain', 'strains', 'strands', 'strata', 'strategic', 'strategies', 'strategiesthat', 'strategize', 'strategy', 'stratigraphy', 'strauss', 'strawberries', 'strawser', 'stream', 'streaming', 'streamline', 'streamlined', 'streamlining', 'streams', 'street', 'streeter', 'strength', 'strengthen', 'strengthened', 'strep', 'stress', 'stresses', 'stressful', 'strict', 'strike', 'string', 'stringent', 'strives', 'stroke', 'stromal', 'strong', 'strongly', 'struck', 'structural', 'structure', 'structured', 'structures', 'struggling', 'struts', 'sttr', 'stu', 'student', 'studentfox', 'studentlowell', 'studentpurdue', 'students', 'studentslisbon', 'studentsskills', 'studied', 'studies', 'studiesamerican', 'studiescolby', 'studiesdaiwa', 'studiesdean', 'studiesteach', 'studiestissue', 'studieswarren', 'studio', 'studios', 'study', 'studying', 'studyjohn', 'stx', 'style', 'styling', 'stylized', 'sub', 'subarray', 'subcloning', 'subcommittee', 'subcontractors', 'subcutaneous', 'subdivision', 'subject', 'subjects', 'sublime', 'submission', 'submissions', 'submit', 'submittal', 'submittals', 'submitted', 'submitting', 'subordinates', 'subs', 'subsampling', 'subsequent', 'subsequently', 'subsidiary', 'subspecies', 'substance', 'substances', 'substantial', 'substantially', 'substitute', 'substrates', 'subsurface', 'subtraction', 'subunit', 'suburban', 'subway', 'success', 'successes', 'successful', 'successfully', 'succession', 'successor', 'succinctly', 'sucre', 'sudbury', 'suddenly', 'sue', 'sugaring', 'suggest', 'suggested', 'suggestion', 'suggestions', 'suicidal', 'suicide', 'suis', 'suit', 'suitability', 'suitable', 'suite', 'suites', 'sulfide', 'sum', 'sumitomo', 'summaries', 'summarize', 'summarizing', 'summary', 'summarydesk', 'summer', 'summerland', 'summers', 'sumoy', 'sun', 'sunquest', 'sunshine', 'suny', 'super', 'superconductors', 'superfund', 'superior', 'superiors', 'superlattice', 'supervise', 'supervised', 'supervises', 'supervising', 'supervision', 'supervisor', 'supervisors', 'supervisorstate', 'supervisory', 'supplemental', 'supplier', 'suppliers', 'supplies', 'suppliessection', 'supply', 'supplysystems', 'support', 'supportavionics', 'supported', 'supporting', 'supportive', 'supporto', 'supports', 'suppressed', 'suppresser', 'suppression', 'sure', 'surface', 'surfaces', 'surgery', 'surgical', 'surpassed', 'surpassing', 'surprises', 'surrey', 'surrounding', 'surveillance', 'survey', 'surveyed', 'surveying', 'surveyors', 'surveyoru', 'surveys', 'surveysvisiting', 'survival', 'surviving', 'susan', 'susceptibility', 'suspended', 'suspension', 'suss', 'sustainability', 'sustainable', 'sustaining', 'sutter', 'suzuki', 'svensson', 'sverko', 'svg', 'svm', 'svn', 'swaminathan', 'swamp', 'sweat', 'swiftwater', 'swim', 'swimmer', 'swimming', 'swing', 'switch', 'switchboard', 'switches', 'switzerland', 'syblîâ', 'sydney', 'sydorenko', 'syllabi', 'syllabus', 'sylvatic', 'symbian', 'symbiont', 'symonds', 'symondsessex', 'sympathetic', 'symphony', 'symposia', 'symposium', 'symptoms', 'synapse', 'sync', 'synergies', 'synopsis', 'syntax', 'synthesis', 'synthesized', 'synthesizeknown', 'synthesizing', 'synthetasethe', 'synthetic', 'synuclein', 'syrup', 'sysadmin', 'systematic', 'systematics', 'systems', 'systemsboston', 'systemso', 'szwenia', 'tables', 'tablet', 'tablets', 'tabs', 'tabulate', 'tabulation', 'tachira', 'tacking', 'tackle', 'tactful', 'tactical', 'tactics', 'tadpole', 'taft', 'tag', 'tagged', 'tailed', 'tailor', 'tailored', 'tailoring', 'takemedical', 'taken', 'taking', 'talent', 'talented', 'talents', 'tam', 'tamil', 'tamoxifen', 'tampa', 'tamworth', 'tan', 'tank', 'tanks', 'tanner', 'tanzania', 'taped', 'tar', 'target', 'targeting', 'targets', 'tarraz', 'task', 'tasking', 'tasks', 'taskscarried', 'taskswilling', 'taskswork', 'tat', 'taught', 'tauroursodeoxy', 'tax', 'taxa', 'taxonomic', 'taxonomy', 'taylor', 'tca', 'tcar', 'tcbs', 'tcl', 'tcm', 'tcp', 'tcpdump', 'tcz', 'tdd', 'tds', 'teach', 'teacher', 'teacherclear', 'teacherharwood', 'teacherlife', 'teacherlong', 'teachermiddle', 'teachermilton', 'teachers', 'teaching', 'team', 'teammates', 'teamo', 'teamregional', 'teams', 'teamwork', 'tear', 'tec', 'tech', 'technical', 'technically', 'technician', 'technicianclean', 'techniciandr', 'technicianibm', 'technicianlms', 'technicianmote', 'technicianpizzo', 'technicians', 'technicianstate', 'technicianthe', 'technicianthree', 'technicianu', 'technics', 'technique', 'techniques', 'technolgy', 'technological', 'technologies', 'technologist', 'technologists', 'technologistu', 'technology', 'technologyanna', 'technologyinter', 'technologynem', 'technologystate', 'technologyuniv', 'technophile', 'techs', 'techsound', 'tecnologia', 'teh', 'tek', 'telecom', 'teleconference', 'telemetry', 'telephone', 'telephones', 'telephony', 'telescopes', 'television', 'tellercitizens', 'tellers', 'tem', 'temperate', 'temperature', 'temperatures', 'template', 'templates', 'tempo', 'temporally', 'temporary', 'tendon', 'tendons', 'tennessee', 'tennis', 'tens', 'tense', 'tensorflow', 'tentative', 'tenure', 'terabytes', 'teradyne', 'term', 'terminal', 'terminals', 'terminate', 'termination', 'terminations', 'terms', 'terra', 'terrain', 'terrasync', 'territories', 'territory', 'test', 'tested', 'testengineer', 'tester', 'testimony', 'testing', 'testingresearch', 'testmachine', 'tests', 'tethered', 'tetra', 'text', 'textbook', 'textbooks', 'texture', 'tga', 'thank', 'thatincluded', 'thaw', 'thayer', 'theater', 'theatrix', 'thecompany', 'thedevelopment', 'theimenti', 'thematic', 'theoretical', 'theory', 'theorysystem', 'thepantone', 'ther', 'therapeutic', 'therapeutics', 'therapies', 'therapist', 'therapistnew', 'therapists', 'therapy', 'therapycenter', 'theresa', 'thermal', 'thermo', 'thermodynamic', 'thermodynamics', 'thermofluids', 'thermometers', 'theses', 'thesis', 'thesludge', 'thesun', 'theta', 'thframingham', 'thickness', 'thimphu', 'things', 'thinker', 'thinking', 'thinks', 'thirds', 'thirteen', 'thirty', 'thisprocess', 'thisthree', 'thomson', 'thon', 'thornwood', 'thorough', 'thought', 'thousands', 'threaded', 'threading', 'threatened', 'threshold', 'thresholding', 'thrives', 'throated', 'throughput', 'thrown', 'thrush', 'thrushes', 'thrust', 'thruster', 'thrusts', 'thymeleaf', 'tibiofemoral', 'tica', 'tick', 'ticketing', 'tickets', 'ticks', 'tied', 'tier', 'tierisabella', 'tight', 'tile', 'till', 'timber', 'timberland', 'time', 'timecards', 'timekeeping', 'timeline', 'timelines', 'timely', 'timemaritime', 'times', 'timeîâ', 'timing', 'tiner', 'tire', 'tissue', 'tissues', 'titanium', 'title', 'titled', 'titles', 'titration', 'titrations', 'tkn', 'tlc', 'tnb', 'tncc', 'tnpost', 'toad', 'toads', 'todistribute', 'toe', 'toestablish', 'tof', 'toilet', 'toilets', 'tolerance', 'tolerances', 'tolerancing', 'tolerant', 'tolland', 'tom', 'tomatoes', 'tomcat', 'tomography', 'ton', 'tone', 'took', 'tool', 'tooling', 'toolkit', 'tools', 'toolso', 'topics', 'topography', 'topotherapy', 'topsfield', 'topsham', 'toresolve', 'torque', 'torro', 'torseptember', 'tortoise', 'tortoises', 'total', 'totals', 'tough', 'tour', 'tourism', 'tournaments', 'tours', 'towereducationm', 'towers', 'town', 'towns', 'toxic', 'toxicants', 'toxicity', 'toxicological', 'toxicologist', 'toxicologists', 'toxicology', 'toxicologyuniv', 'toxin', 'tpm', 'tpmsmart', 'tps', 'trace', 'traceability', 'tracers', 'tracing', 'track', 'tracked', 'tracker', 'trackers', 'tracking', 'trackingo', 'trackingthis', 'tract', 'traction', 'tractor', 'trade', 'tradeand', 'traded', 'tradeoffs', 'tradeshows', 'tradition', 'traditional', 'traditionally', 'traf', 'traffic', 'trail', 'trailer', 'trailering', 'trailhttp', 'trails', 'train', 'trained', 'trainees', 'traineeship', 'trainer', 'trainers', 'training', 'traininghighly', 'trainings', 'trait', 'tran', 'transaction', 'transactions', 'transcribed', 'transcript', 'transcriptional', 'transcriptomic', 'transducer', 'transducers', 'transduction', 'transects', 'transfection', 'transfer', 'transform', 'transformation', 'transformed', 'transgenic', 'transhelburne', 'transistor', 'transit', 'transition', 'transitional', 'transitionapril', 'transitioned', 'transitioning', 'translate', 'translated', 'translating', 'translation', 'translational', 'translators', 'transmission', 'transmits', 'transmitters', 'transmitting', 'transport', 'transportation', 'transporting', 'transverse', 'trap', 'trapped', 'trapping', 'traps', 'trauma', 'travel', 'traveled', 'traveler', 'traxpro', 'trc', 'treat', 'treated', 'treating', 'treatment', 'treatments', 'tree', 'trees', 'trenbolone', 'trend', 'trends', 'trent', 'trenton', 'tri', 'triage', 'trial', 'trials', 'triatoma', 'triatominae', 'triatomine', 'tribal', 'tribe', 'tribes', 'trickling', 'tried', 'trimble', 'trip', 'tripled', 'tripwire', 'trk', 'trna', 'troller', 'trombly', 'tropical', 'trouble', 'troubleshoot', 'troubleshooter', 'troubleshooting', 'troubleshoots', 'troubleshot', 'trout', 'trove', 'troy', 'truck', 'trucks', 'trust', 'trusted', 'trustees', 'trypanosoma', 'trypanosomes', 'trypsinization', 'tsh', 'ttc', 'ttg', 'tube', 'tubes', 'tucson', 'tudca', 'tuesday', 'tuitions', 'tulane', 'tullahoma', 'tulsa', 'tumor', 'tumorigenesis', 'tumors', 'tuned', 'tunel', 'tunneling', 'turbid', 'turbidity', 'turbine', 'turbochargers', 'turbomachinery', 'turbomachines', 'turbuhalerî', 'turf', 'turn', 'turning', 'turnover', 'turtles', 'tutor', 'tutored', 'tutorial', 'tutorials', 'tutoring', 'tutors', 'tutoruniversity', 'tutorwilliston', 'twice', 'twitter', 'type', 'types', 'typha', 'typical', 'typically', 'typing', 'typist', 'uav', 'ubuntu', 'ucinet', 'ucr', 'ude', 'udp', 'ueet', 'uiallthings', 'uiq', 'ukraine', 'ulala', 'ultimate', 'ultra', 'ultrasonic', 'ultrasound', 'umass', 'uml', 'unacceptable', 'unauthorized', 'unavailable', 'unbiased', 'unburned', 'uncertainty', 'unclipping', 'uncluttered', 'unctad', 'underestimated', 'underflow', 'undergoing', 'undergrad', 'undergraduate', 'undergraduates', 'undergraduation', 'underground', 'underhill', 'underlying', 'understand', 'understandable', 'understanding', 'understood', 'undertake', 'undertaking', 'underwater', 'undocumented', 'unemployment', 'unexpected', 'unexpectedly', 'unexplored', 'unh', 'unifier', 'uniform', 'unilever', 'unimportant', 'union', 'unique', 'unit', 'unite', 'united', 'units', 'univ', 'univariate', 'univeristy', 'universal', 'universidad', 'universitaire', 'universitario', 'universities', 'university', 'universitydeans', 'universitymay', 'unix', 'unixsoftware', 'unknown', 'unknowns', 'unloading', 'unmanned', 'unmapped', 'unparalleled', 'unplug', 'unprecedented', 'unsaturated', 'unscented', 'unsolved', 'unsteadygas', 'unsupervised', 'unsure', 'untangling', 'untimely', 'unum', 'upbeat', 'upcoming', 'update', 'updated', 'updates', 'updating', 'upgrade', 'upgraded', 'upgradeddesign', 'upgrades', 'upgrading', 'upholt', 'upinformational', 'upkeep', 'upper', 'ups', 'upselling', 'upset', 'upstream', 'uptake', 'urban', 'urbano', 'ureca', 'urinalysis', 'urine', 'urop', 'usa', 'usability', 'usable', 'usaf', 'usage', 'usauniversity', 'usda', 'use', 'usedaseptic', 'usedcomputer', 'usedcritical', 'usedeveloped', 'usedleadership', 'usedteamwork', 'usedwhile', 'useeducationm', 'useful', 'usepa', 'user', 'users', 'uses', 'usfs', 'usfws', 'usgcb', 'usgs', 'usp', 'ust', 'ustainable', 'usually', 'uterine', 'utilities', 'utility', 'utilization', 'utilize', 'utilized', 'utilizes', 'utilizing', 'uttar', 'uva', 'uvm', 'uwc', 'vaassistant', 'vaassociate', 'vaauthorized', 'vacant', 'vacation', 'vaccinated', 'vaccination', 'vaccine', 'vaccines', 'vacuum', 'vaeducationph', 'valet', 'valetcountry', 'valhalla', 'validate', 'validated', 'validates', 'validating', 'validation', 'validations', 'validity', 'valley', 'valleys', 'valuable', 'valuation', 'value', 'valued', 'values', 'valve', 'valves', 'van', 'vanasse', 'vance', 'vancouver', 'vanderhyden', 'vanderveer', 'vapor', 'variability', 'variable', 'variables', 'variance', 'variances', 'variant', 'variants', 'variation', 'varied', 'varieties', 'variety', 'varsity', 'vary', 'varying', 'vascular', 'vaseva', 'vasodilation', 'vaulter', 'vba', 'vch', 'vdh', 'vector', 'vectors', 'vegasactivities', 'vegetable', 'vegetation', 'vegetative', 'vehicle', 'vehicles', 'velocity', 'vender', 'vendor', 'vendors', 'venture', 'ventures', 'venues', 'verbal', 'verde', 'vergennes', 'verification', 'verified', 'verify', 'verifying', 'verizon', 'verlag', 'vermont', 'vermontan', 'vermontmay', 'vermontnorthern', 'vermontteaching', 'vermontwildlife', 'vernal', 'verona', 'versatile', 'verse', 'versed', 'version', 'versioned', 'versioning', 'vertebrate', 'vessel', 'vessels', 'veteran', 'veterans', 'veterinarian', 'veterinary', 'vhb', 'viability', 'viable', 'viasat', 'vibration', 'vibrio', 'vibrios', 'vice', 'victoria', 'vidana', 'video', 'videos', 'view', 'viewcontent', 'viewed', 'viewer', 'viewpoints', 'views', 'vigorously', 'vijay', 'village', 'vimovo', 'vincent', 'vining', 'vintnerraven', 'violation', 'violations', 'violators', 'violence', 'violent', 'violet', 'vip', 'viral', 'vireos', 'virgin', 'virginia', 'virology', 'virtual', 'virtualization', 'virulence', 'virus', 'viruses', 'vis', 'visas', 'visible', 'visio', 'vision', 'visit', 'visited', 'visiting', 'visitors', 'visits', 'viso', 'vista', 'visual', 'visualization', 'visualizations', 'visuals', 'vital', 'vitamins', 'vitek', 'vitro', 'vivo', 'vlanso', 'vlsi', 'vmd', 'vmobile', 'vocabulary', 'vocalizations', 'voice', 'void', 'vol', 'volans', 'volatile', 'volksenior', 'voltage', 'volume', 'volumes', 'voluntary', 'volunteer', 'volunteered', 'volunteerism', 'volunteers', 'volunteeru', 'von', 'voucher', 'vouchers', 'vparticipating', 'vpn', 'vpr', 'vtaas', 'vtadditional', 'vtathens', 'vtburlington', 'vtcommunity', 'vtcustom', 'vtdatabase', 'vtgis', 'vtgraphic', 'vtk', 'vtskillstyping', 'vtunderhill', 'vtwork', 'vulnerabilities', 'vulnerability', 'vulnificus', 'wachovia', 'wading', 'wafer', 'wafers', 'waitress', 'waitsfield', 'waitstaff', 'walk', 'walked', 'walks', 'wall', 'walled', 'walls', 'walnut', 'walter', 'waltham', 'wanee', 'wang', 'want', 'wanted', 'wants', 'warblers', 'warburton', 'ware', 'warehouse', 'warehouses', 'warm', 'warped', 'warping', 'warrantee', 'warranties', 'warren', 'warrior', 'warwick', 'wash', 'washers', 'washing', 'washington', 'wasinstrumental', 'wasorganized', 'waste', 'wastes', 'wastestate', 'wastewater', 'watch', 'watchdog', 'watched', 'water', 'waterbury', 'watercolors', 'waterfall', 'waterfalls', 'waterfowl', 'waterloo', 'waterlooaps', 'waterquality', 'waters', 'watershed', 'watersheds', 'watersports', 'waterville', 'watson', 'wave', 'wavefront', 'way', 'wayne', 'ways', 'weapons', 'wearable', 'weather', 'weaver', 'web', 'webex', 'webinar', 'webinars', 'weblog', 'weblogic', 'webmastergreen', 'webpage', 'website', 'websitehelped', 'websites', 'websitevermont', 'websphere', 'webstorm', 'weebly', 'week', 'weekend', 'weekends', 'weeklaborer', 'weekly', 'weeks', 'weekthis', 'wefmsp', 'weiden', 'weighed', 'weighing', 'weight', 'weights', 'weinheim', 'weirs', 'welcoming', 'welded', 'weldment', 'welds', 'welfare', 'wellness', 'wells', 'wendy', 'went', 'west', 'westborough', 'western', 'westerns', 'westfield', 'westford', 'westminster', 'wet', 'wetland', 'wetlands', 'wetlandsweb', 'whale', 'whales', 'whatwe', 'wheat', 'wheelock', 'whileminimizing', 'whileopening', 'whistler', 'white', 'whitney', 'whitters', 'wichita', 'wide', 'wife', 'wiki', 'wild', 'wilder', 'wilderness', 'wildfire', 'wildfires', 'wildland', 'wildlife', 'wildt', 'wiley', 'wilkes', 'william', 'williamsburg', 'williamson', 'willimantic', 'willing', 'willis', 'williston', 'willits', 'willow', 'wiloughby', 'wilson', 'win', 'winchester', 'wind', 'window', 'windows', 'windsor', 'wine', 'winery', 'wines', 'wings', 'winner', 'winning', 'winooski', 'winooskiserved', 'wins', 'winter', 'winthrop', 'wipes', 'wireframes', 'wireless', 'wiring', 'wisconsin', 'wish', 'wistar', 'wit', 'withcanyon', 'withclinical', 'withconfidence', 'witheuropean', 'withhigh', 'witness', 'wojcik', 'wojcikit', 'women', 'wonyoung', 'wood', 'woodinville', 'woodpecker', 'woodpeckers', 'woods', 'woodstock', 'woodworking', 'woody', 'wooster', 'worcester', 'word', 'wordpress', 'wordresearch', 'words', 'work', 'workassistant', 'workbench', 'worked', 'worker', 'workers', 'workflow', 'workflows', 'workforce', 'workfunction', 'workgroups', 'working', 'workload', 'workplace', 'works', 'workshop', 'workshops', 'workspaces', 'workstation', 'world', 'worldwide', 'worth', 'wotus', 'wound', 'wounded', 'wpm', 'wrangling', 'write', 'writer', 'writers', 'writerspss', 'writes', 'writing', 'writingcontact', 'written', 'wrm', 'wrong', 'wrote', 'wrotehta', 'wroteinternal', 'www', 'wwwnc', 'wyoming', 'xactimate', 'xavier', 'xbqoctober', 'xcalibur', 'xccdf', 'xenograft', 'xestospongia', 'xiangtan', 'xiaoli', 'xls', 'xml', 'xoma', 'xpress', 'xps', 'xrd', 'xrî', 'xsd', 'xsl', 'xslt', 'yahoo', 'year', 'yearbook', 'yearfull', 'yearly', 'years', 'yearsesri', 'yearsflinders', 'yearsgraph', 'yearsinvited', 'yearso', 'yearssas', 'yeast', 'yellow', 'yield', 'yielded', 'yielding', 'yields', 'ymca', 'yolanda', 'yore', 'york', 'yorkorganized', 'yorksaint', 'young', 'youngyoung', 'yousef', 'youth', 'yrs', 'zaruk', 'zebra', 'zeldin', 'zeng', 'zengresearch', 'zero', 'zhong', 'zilembo', 'zimecki', 'zip', 'zirconocene', 'zmarch', 'zomig', 'zomigî', 'zone', 'zones', 'zoonotic', 'zosia', 'zudaõ', 'zurima', 'ãæcomputer', 'ètravel', 'ô_torrent']
[[0 0 0 ... 0 0 0]
[0 0 0 ... 0 0 0]
[0 0 0 ... 0 0 0]
...
[0 0 0 ... 0 0 0]
[0 0 0 ... 0 0 0]
[0 0 0 ... 0 0 0]]
Train Naive Bayes Classifier Model¶
[ ]:
X = c
y = resume_df['class']
[ ]:
X.shape
(125, 11319)
[ ]:
y.shape
(125,)
[ ]:
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
X_train, X_test, y_train, y_test = train_test_split(X,y,test_size=0.2)
NB_classifier = MultinomialNB()
NB_classifier.fit(X_train, y_train)
MultinomialNB()
[ ]:
# Predicting the performance on train data
y_predict_train = NB_classifier.predict(X_train)
y_predict_train
cm = confusion_matrix(y_train, y_predict_train)
sns.heatmap(cm, annot = True)
<AxesSubplot:>
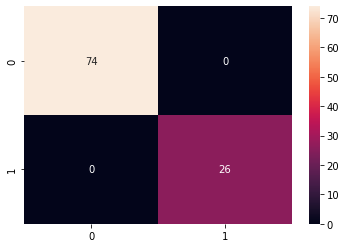
[ ]:
# Predicting the Test set results
y_predict_test = NB_classifier.predict(X_test)
cm = confusion_matrix(y_test, y_predict_test)
sns.heatmap(cm, annot = True)
<AxesSubplot:>
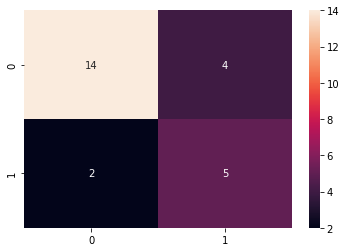
[ ]:
# Question - What happens if you tweak the test-train split?